Hello Python Developers, In this article we are about to see how to print Fibonacci series in Python with the help of various ways including using Python for loop, Python functions, recursion, and dynamic programming.
You must know the Python basic concepts to understand this Fibonacci series example. You can learn all of them from the Python tutorials.
- Python for loop π Click Here
- Python Function π Click Here
- Python If else π Click Here
Let’s begin the actual tutorial.
Headings of Contents
What is Fibonacci series in Python?
Fibonacci series is the series of numbers where each number is the sum of the two preceding numbers. Usually Fibonacci series starts with 0 and 1. To understand in better ways you can see the below picture of the Fibonacci series.
There are several ways to print the Fibonacci series in Python. We will explore different methods to achieve this and also we will discuss their implementations and efficiency.
Let’s begin.
Method 1:- Print Fibonacci Series in Python using For Loop:
In this, we are about to use Python for loop to print the Fibonacci series. Below is the code to generate the Python Fibonacci series.

fibonacci_series = [0, 1] # num is number of items appear in fibonacci series num = 5 # Iterating from 2 to till minus 1 to num and each iteration we calculating the fibonacci series and append into # fibonacci_series for i in range(2, num): fibonacci_series.append(fibonacci_series[-1] + fibonacci_series[-2]) print("Fibonacci Series is:- ", fibonacci_series)
Output
Fibonacci Series is:- [0, 1, 1, 2, 3]
Explanation
- First, we have defined a list that contains the first two items of the Fibonacci series that is 0 and 1.
- Defined a num variable with a 5 value and this value indicates how many items will be available in the Fibonacci series. Here you can input from users as well.
- At each iteration, we calculate the next Fibonacci number by adding the last two numbers in the series and appending it to the list.
- And finally print the Fibonacci series by printing the fibonacci_series.
Method 2:- Print Fibonacci Series in Python using Function:
To print the Fibonacci series in Python using the function, you need to just put the above code inside the function pass num as a parameter in the Python function, and return the Fibonacci series.
You can learn Python functions from here.
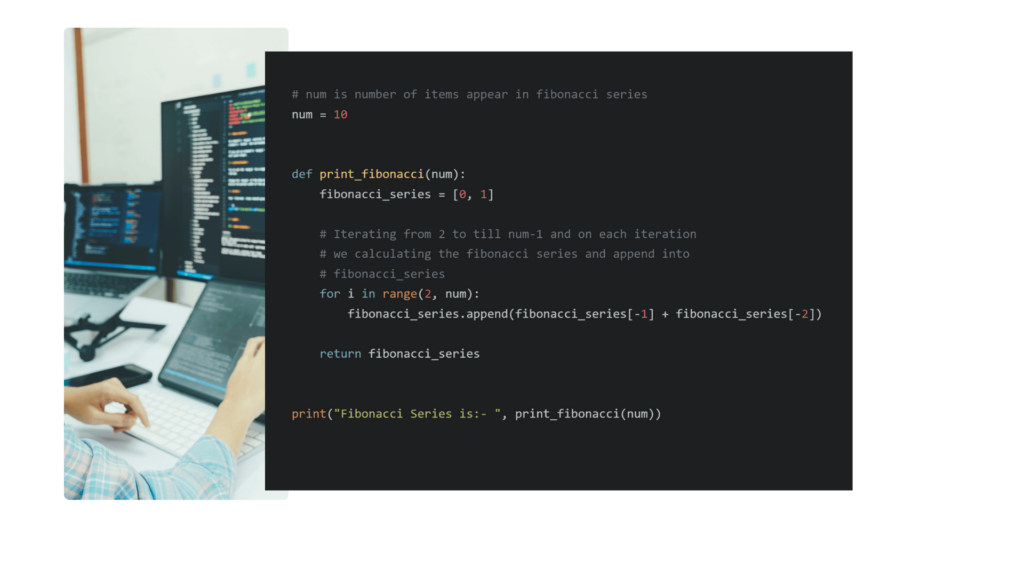
# num is number of items appear in fibonacci series num = 10 def print_fibonacci(num): fibonacci_series = [0, 1] # Iterating from 2 to till minus 1 to num and each iteration we calculating the fibonacci series and append into # fibonacci_series for i in range(2, num): fibonacci_series.append(fibonacci_series[-1] + fibonacci_series[-2]) return fibonacci_series print("Fibonacci Series is:- ", print_fibonacci(num))
Output will be:- Fibonacci Series is:- [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Let’s break down the above code.
- First, we have defined a Python function and it takes num as a parameter where num indicates the number of items that will appear in the Fibonacci series.
- Second, we have defined a list that contains the first two items of the Fibonacci series, 0 and 1.
- Defined a num variable with the 5 value and this value indicates how many items will be available in the Fibonacci series. Here you can take input from users as well.
- At each iteration, we calculate the next Fibonacci number by adding the last two numbers in the series and appending it to the list.
- After appending all the items of the Fibonacci series, I have returned the fibonacci_series list from the Python function.
- And finally print the Fibonacci series by printing the fibonacci_series.
Method 3:- print fibonacci series in Python using recursion:
Recursion is the most popular method to generate the Fibonacci series, Most of the interviewers asked to generate the Fibonacci series Python recursion.
Recursion is a kind of Python function that calls itself again and again. Let’s print the Fibonacci series in Python using recursion.
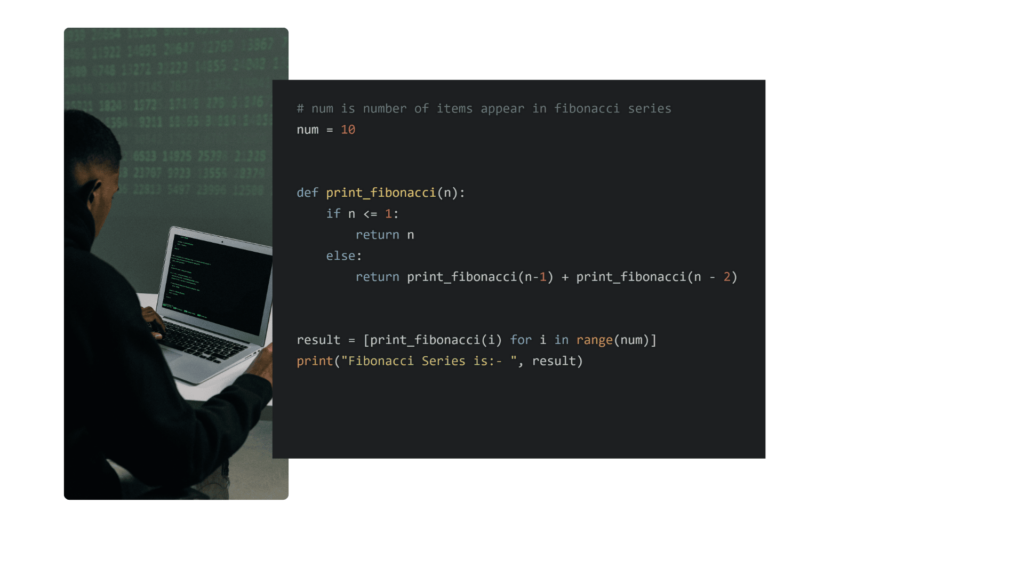
# num is number of items appear in fibonacci series num = 10 def print_fibonacci(n): if n <= 1: return n else: return print_fibonacci(n-1) + print_fibonacci(n - 2) result = [print_fibonacci(i) for i in range(num)] print("Fibonacci Series is:- ", result)
The Output would be
Fibonacci Series is:- [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Code Explanation:
- The function print_fibonacci() takes an integer num as input and returns the nth Fibonacci number.
- If n is 0 or 1, it returns num (base cases).
- Otherwise, it recursively calls itself with n-1 and n-2 to calculate the Fibonacci number.
- We use list comprehension to generate the Fibonacci series up to the nth term.
Method 4:- print Fibonacci series in Python using dynamic programming:
Dynamic programming in Python is the way of solving complex problems by breaking the complex problems into separate problems solving each problem only once and also storing their result to avoid redundant computations.
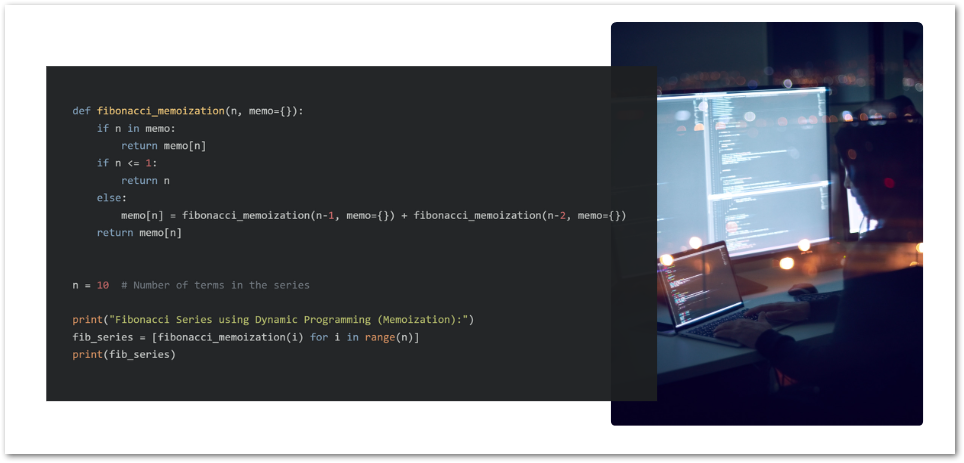
Lelt’s print Fibonacci series with the help of Python dynamic programming.
def fibonacci_memoization(n, memo={}): if n in memo: return memo[n] if n <= 1: return n else: memo[n] = fibonacci_memoization(n-1, memo={}) + fibonacci_memoization(n-2, memo={}) return memo[n] n = 10 # Number of terms in the series print("Fibonacci Series using Dynamic Programming (Memoization):") fib_series = [fibonacci_memoization(i) for i in range(n)] print(fib_series)
The output would be:
Fibonacci Series using Dynamic Programming (Memoization): [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Let’s break down the above code.
- The fibonacci_memoization() function takes an additional parameter memo which is used to store the computed Fibonacci series.
- If the Fibonacci series of n value is already available and computed in the memo, It returns the stored value.
- Otherwise, it will calculate the Fibonacci series of the n value using recursion and store the results in the memo before returning it.
This is how you can write a Python program to find the Fibonacci series.
Conclusion
In today’s article, we have seen how to print Fibonacci series in Python using various ways including using Python for loop, Python function, Python recursion, and Python dynamic programming. You can go with any solution as per your understanding and requirements.
But as a Python developer, You must know all the methods, and even you can tell to Python interviewer about all the approaches to implement them.
If you found this article helpful, please share and keep visiting for further Python interesting tutorials.
Thanks for your timeβ¦