In this Python article, we will see how to convert a Python List to CSV with the help of the example. Python provides a built-in nodule that is used to perform CSV-related operations like reading the CSV file or writing the CSV files with the help of some lines of code.
Python CSV module is a built-in module which means you don’t need to install it in your Python environment, just import it using the import
keyword and start using it.
In real-life Python applications, sometimes we are required to convert a simple Python list to a CSV file or a List of lists to a CSV file, at that time we get stuck but after this article, you will be completely able to convert any type of List to a CSV file.
Let’s see some examples to write a Python List to a CSV file with the help of the Python CSV module.
Headings of Contents
Convert a Python List to a CSV file
Here, we will see multiple types of lists like lists of one item, nested lists of lists, etc to convert into a CSV file using the Python CSV module.
Convert a List of single items into a CSV File
If you have a Python list that has only single items, not sublists then you can use below below-mentioned Python code to convert it into a CSV file.
import csv language = ['Python', 'Java', 'PHP', 'C', 'C++', 'R'] header = ['Name'] filename = "languages.csv" with open(filename, "w", newline="") as file: writer = csv.writer(file) writer.writerow(header) for item in languages: writer.writerow([item])
In the above code:
- We import the
CSV
module. - We define a
language
andheader
list wherelanguage
contain the items and theheader
list contains the header row. - We define a variable
filename
that contains the name of the file where csv will be saved. - Before writing the data, we use
writer.writerow(header)
to write the header row to the CSV file. - Then, we iterate over each item in the data list and write them as separate rows.
- We open the filename ‘language.csv‘ using the
open()
function in the write mode (‘w’). - Create a write object using
csv.writer()
method by passing the file into it. - As you can see, before writing the
language
list data we use thewriter.writerow(header)
to write the header to a CSV file. - Finally, we have iterated each item of the
language
list and wrote it into a separate row of the CSV file usingwriter.writerow([item])
.
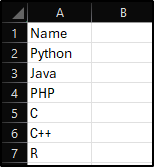
How to Write List of Lists into CSV File
Here, we have a Python nested list and the nested list represents the information of of the students like student name, department, and their salary, now we are about to convert the nested list into a CSV file with the help of the Python CSV module.
Let’s see:
import csv student = [ ["Vishvajit", "IT", 20000], ["Harsh", "Digital Marketing", 15000], ["Vinay", "IT", 12000], ["Mohak", "Marketing", 18000], ["Natasha", "IT", 220000], ] header = ['Name', 'Department', 'Salary'] filename = "students.csv" with open(filename, "w", newline="") as file: writer = csv.writer(file) writer.writerow(header) writer.writerows(students)
In the above example:
- We import the
CSV
module. - We define
student
andheader
list where thestudent
list contains the student’s information like name, department, and salary, and aheader
list containing the header row. - We define a variable
filename
that contains the name of the file where CSV will be saved. - Before writing the data, we use
writer.writerow(header)
to write the header row to the CSV file. - Then, we iterate over each item in the data list and write them as separate rows, just like before.
- We open the filename ‘students.csv‘ using the
open()
function in the write mode (‘w’). - Create a write object using
csv.writer()
method by passing the file into it. - As you can see, before writing the
student
list data we use thewriter.writerow(header)
to write the header to a CSV file. - Finally, We pass the students to
writer.writerows()
to write all the nested lists to a CSV file.

This is how you can convert a Python nested list to a CSV file using a Python CSV module.
Convert List of Dictionaries into CSV File
If you have a list of Python dictionaries then you need to change a little bit of code in the above code.
Here, You can see the modified code.
import csv # Example list of dictionaries data = [ {'Name': 'John', 'Age': 30, 'Location': 'New York'}, {'Name': 'Alice', 'Age': 25, 'Location': 'Los Angeles'}, {'Name': 'Bob', 'Age': 35, 'Location': 'Chicago'} ] # Header for the CSV file header = ['Name', 'Age', 'Location'] # Path to save the CSV file csv_file_path = 'students.csv' # Write data to CSV file with open(csv_file_path, 'w', newline='') as csvfile: writer = csv.DictWriter(csvfile, fieldnames=header) # Write the header writer.writeheader() # Write data for row in data: writer.writerow(row)
In the above code:
data
is a list of dictionaries where each dictionary represents a row in the CSV file.header
is a list containing the keys of the dictionaries, which will be used as the header row in the CSV file.- We open the CSV file in write mode and create a
csv.DictWriter
object, specifying thefieldnames
as the header. - We use
writer.writeheader()
to write the header row to the CSV file. - Then, we iterate over each dictionary in the data list and write them as rows using
writer.writerow(row)
.
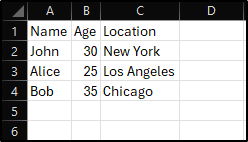
This is how you can convert a list of dictionaries into a CSV file using the Python CSV module.
Useful Python Articles
Conclusion
So, throughout this article, we have seen how we can convert a Python list to a CSV file with the help of the Python built-in module called CSV.
You can convert any kind of list like a list of single items, nested lists, and a list of dictionaries into a CSV file with some line of code.
If you found this article helpful, please share and keep visiting for further interesting tutorials.
Thanks for reading….