Hello Python geeks, In this guide, we are going to learn all about types of inheritance in Python along with examples.
Inheritance is the most important part of Object-Oriented Programming. If you are not familiar with Python inheritance, Then I will recommend you, please read our previous Python inheritance tutorial and then continue it.
In this guide, we will see all about types of inheritance in Python using various examples, so that you can clear with types of inheritance in Python.
Before going through this article, we will see a brief description of Python inheritance. To understand this article, you should have knowledge of Python class and object.
Headings of Contents
Python Inheritance
Inheritance in Python provides a facility to create a new class (drived class or child class ) using another class (base class or parent class ). The Newly created class has the authority to access or inherit all the properties and methods from the existing class.
Inheritance is basically used for reusability. That means you don’t need to write the same code again and again.
Here we will see a simple example to understand Python inheritance, and after we will go through types of inheritance in Python.
Example:
class Person:
#Constructor
def __init__(self, first_name, last_name):
self.first = first_name
self.last = last_name
class Student(Person):
#method
def fullName(self):
print(f"My name is {self.first + ' ' + self.last}.")
s1 = Student('Vishvajit', 'Rao')
s1.fullName()
Output
My name is Vishvajit Rao.
In above example, we have created a class Student using Person. Student class inherit all the properties ( first and last ) from the Person class.
Let’s see Types of Inheritance in Python one by one along with examples.
Types of Inheritance Python
There are four types of Inheritance in Python that are described below using their examples.
Single Inheritance:
Single Inheritance in Python allows the derived class to inherit all the properties and methods from the single parent class.
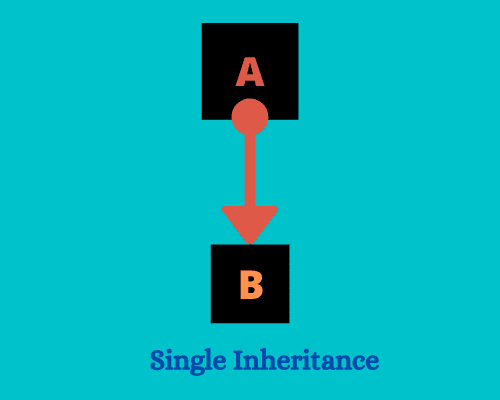
Example:
class Computer:
#Constructor
def __init__(self, name):
self.laptop_name = name
class Laptop(Computer):
#Method to find laptop name
def Name(self):
print(f'laptop name is:- {self.laptop_name}')
l1 = Laptop('Lenovo')
l1.Name()
l2 = Laptop('HP')
l2.Name()
Output
laptop name is:- Lenovo
laptop name is:- HP
Multiple Inheritance:
Multiple Inheritance in Python allows a class can be derived from more than one class. This type of class is called multiple inheritances.
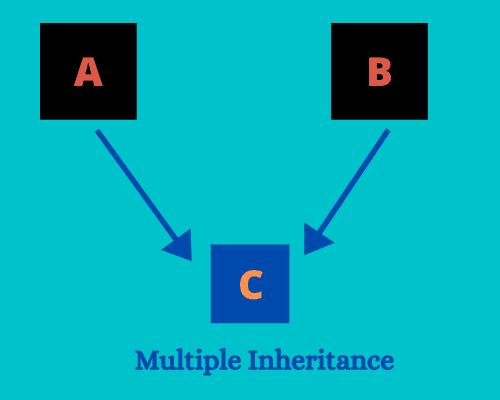
Example:
class Father:
#Constructor
def __init__(self, fname):
self.father_name = fname
class Mother:
#Constructor
def __init__(self, mname):
self.mother_name = mname
class Child(Father, Mother):
def __init__(self, fname, mname):
Father.__init__(self,fname)
Mother.__init__(self,mname)
def Parent(self):
print(f"My father name is:- {self.father_name}\nMy mother name is:- {self.mother_name}")
c1 = Child('John', 'Ammy')
c1.Parent()
Output
My father name is:- John
My mother name is:- Ammy
Multilevel Inhritance:
Multilevel inheritance in Python allows us to create a child class or derived class using another child class or derived class. Basically in Multilevel inheritance, each derived class will be the parent class of another.
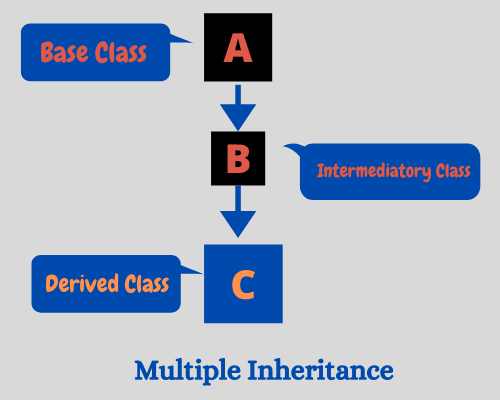
Example:
class Grandfather:
def __init__(self, grandfathername):
self.grandfather_name = grandfathername
class Father(Grandfather):
def __init__(self, fathername, grandfathername):
self.father_name = fathername
Grandfather.__init__(self, grandfathername)
class Son(Father):
def __init__(self, myname, fathername, grandfathername):
self.my_name = myname
Father.__init__(self, fathername, grandfathername)
def myDetails(self):
print(f"My Name is:- {self.my_name}")
print(f"My Father is:- {self.father_name}")
print(f"My Grandfather is:- {self.grandfather_name}")
s1 = Son('Vishvajit', 'Rajesh', 'ABC')
s1.myDetails()
Output
My Name is:- Vishvajit
My Father is:- Rajesh
My Grandfather is:- ABC
Hierarchical Inheritance:
Hierarchical inheritance in Python allows us to create more than one derived class from using one single base class. This type of inheritance is called hierarchical inheritance.

Example:
class Parent:
def func1(self):
print("This is the base class")
class Child1(Parent):
def func2(self):
print("This is the child1 class")
class Child2(Parent):
def func3(self):
print("This is child2 class")
c1 = Child2()
c1.func3()
c1.func1()
print("---------")
c1 = Child1()
c1.func2()
c1.func1()
Output
This is child2 class
This is the base class
---------
This is the child1 class
This is the base class
Hybrid Inheritance:
Inheritance consisting multiple type of inheritance is called hybrid inheritance.
Example:
class Parent:
def func1(self):
print("This is the base class")
class Child1(Parent):
def func2(self):
print("This is the child1 class")
class Child2(Parent):
def func3(self):
print("This is child2 class")
class Child3(Child1, Child2):
def func4(self):
print("This is child4 class")
class Child4(Child1):
def func5(self):
print("This is child5 class")
c1 = Child2()
c1.func3()
c1.func1()
print("---------")
c2 = Child1()
c2.func2()
c2.func1()
print("------")
c3 = Child3()
c3.func4()
c3.func1()
c3.func3()
print("------")
c4 = Child4()
c4.func5()
c4.func2()
Output
This is child2 class
This is the base class
---------
This is the child1 class
This is the base class
------
This is child4 class
This is the base class
This is child2 class
------
This is child5 class
This is the child1 class
Conclusion
So, In this article, you have learned all about types of inheritance in Python along with examples. I hope you don’t have any confusion regarding the types of Python inheritance.
Python inheritance is the most important concepts of Object-Oriented Programming, so if you want to learn OOPs in Python, Then you can’t ignore this.
If this article is helpful for you, please share and keep visiting for further knowledgeable Python tutorials.
For more information:- Click Here
Python OOPs
FAQs about Types of Inheritance in Python
How many types of inheritance in Python?
Ans:- There are four types of Inheritance in Python available:
1. Single Inheritance
2. Multiple Inheritance
3. Multilevel Inheritance
4. Hierarchical Inheritance
What is Inheritance in Python?
Ans:- Inheritance in Python allows creating a new class using another class.
What is __init__ Python?
Ans:- __init__ method is a special method in Python that is automatically called when the object of the class is created.
What is the super() method in Python?
Ans:- The super() function is a built-in function that is used to give access to properties and methods of a child or parent class.
What is single inheritance in Python?
Ans:- Single inheritance in Python allows to create of a new class using a single class.
What is multiple inheritance in Python?
Ans:- Multiple inheritances in Python allows creating of more than one class using an only a single base or parent class.
I hope you have cleared will types of inheritance in Python.
Thanks for your valuable timeΒ πππ