Hi Django developers, In this Django tutorial we are going to learn all about What is Django models and its usages. Model in Django is one of the most important concepts because it plays a major role especially when you are going to develop a database-driven application.
I highly recommend you to learn Python first or you should have basic knowledge of Python programming because Django totally stands on Python. You can master your Python skills by following our free Python programming tutorial.
Important 👉:- How to create an app in Django application
Headings of Contents
What is Django Model?
Django model is a built-in feature that comes with Django by default it is basically a single, definitive source of information about the data that you are storing.
These are some important points about the model in Django.
- Each Django model represents the Python class.
- Each Django model is a sub-class of
django.db.models.Model
class. - Each Django model is mapped to a single database table in SQL Database.
- Each field of the Django model represents the column name in a database table.
- Django gives you an automatically generated database abstraction API to work with Django models like creating an object, deleting an object, updating an object, and getting the object.
As you can see below diagram, what a Django model looks like in SQL ( Structure Query language ) Database.

Django Model ➡️
Django Model Fields and Options
The field is most important in the Django model because without a field you can create a model in Django. The field represents the data type of the Django model fields.
Each field in your Model should be an instance of a Field class.
- AutoField():- This is a primary key field that will automatically be added to your model if you don’t specify it explicitly.
- BigAutoField():- It is a 64-bit bi integer field that fits with an integer from 1 to 9223372036854775807.
- BinaryField():- It is a binary field that stores binary values.
- BooleanField():- A boolean field that stores only True or False at a time.
- CharField():- A string field that stores string from small to large.
- DateField():- It is the date field that represents the Python DateTime.date.
- DateTimeField():- It is the date and time field that is an instance of DateTime.DateTime.
- DecimalField():- It is a decimal field that is mainly used to store the decimal value. It is a fixed precision decimal number.
- DurationField():- It is a field that is used to store the period of time.
- EmailField():- It is an email field that is used to store the email and also it is capable for validate the email using Emailvalidator.
- FileField():- It is a field that is used to upload the file.
- FloatField():- It is a floating point number that represents the instance of the Python float instance.
- ImageField():- It is Image files that are used to store the image and also inherit all the properties and methods from the FieldField() class and also validate whether an image is valid or not.
- IntegerField():- It is a field that is used to store the only integer value.
- JSONField():- A field that is used to store JSON encoded data.
- SlugField():- It is a field that is used to store the URL or slug which is the combination of words, letters,s, and numbers.
- SmallAutoField():- It is a field that is used to store the values under certain limits. The value should be between 1 to 32767.
- SmallIntegerField():- It is a field that is used to store the values under certain limits. The value should be between –32768 to 32767.
- TextField():- It is a large text field.if you specify max_length then it will automatically reflect Textarea in the auto-generate form field.
- TimeField():- It is a field that is used to store time and time should be an instance of DateTime.time class.
- URLField():- A character field for URL.
- UUIDField():- A field for storing universally unique identifiers.
Relationship Fields
- ForeignKey():- A many-to-one relationship this. ForeignKey() class requires two arguments first is the class to which the model is related and the on_delete option.
- ManyToManyField():- This relationship is mainly used with many-to-many relations.
- OneToOneField():- A one-to-one relationship and this is similar to the ForeignKey relation with unique = True but the reverse side of the relation will only return a single object.
Fields Options
These are some field options that are used with the above fields.
- null: If True, Django will store an empty value as Null in the database. default is False.
- blank: If True, the Django field will be allowed to be blank.
- choice: Choice is used to implement choice options in the field.
- db_column: The name of the database column to use for this field. If it is not given Django will use the field name as a column name.
- db_index: If True, Django will create an index for this field.
- editable: If False. The Field will not display in the admin area and ModelField.
- help_text: The extra help text is to be displayed in the form area.
- primary_key: If True, This field will be the primary key of this model.
- unique: If True then this field will be unique for this model.
- validators: List of validators to run this field.
- verbose_name: A human-readable name for this model.
Using Django Model ( How to use Django Model )
Now it’s time to work with the Django model. Here I am going to see a complete demo to use of Django Model with the help of the example with step by step guide.
Creating Model
To create a model go to the models.py file in your Django app directory. Here I will create a model named Student which has some required fields.
from django.db import models
choice = [
('M', "Male"),
('F', "Female")
]
class Student(models.Model):
first_name = models.CharField(db_column="student_first_name", verbose_name=" Student First Name")
last_name = models.CharField(db_column="student_last_name", verbose_name=" Student Last Name")
email = models.EmailField(verbose_name="Student's email")
gender = models.CharField(verbose_name="Student choice", choices=choice)
roll_no = models.CharField(verbose_name="Student roll number")
course = models.CharField(verbose_name="Course name of the student")
address = models.TextField(verbose_name="Student's address", max_length=150)
def __str__(self):
return f"{self.first_name} {self.last_name}"
Creating Migration
After doing anything in the model like creating a model, deleting the model, add new field in the model, delete an existing field from the model. We need to execute two important commands that are makemigrations and migrate.
- makemigrations:- This command will generate a SQL query on the basis of changes that you have done in your model.
- migrate:- migrate command execute generate SQL query by makemigrations command into the database.
After creating the above model run these two commands.
python manage.py makemigrations
python manage.py migrate
Register Model in Admin Area
To see the model in the Django admin area you have to register your model to the admin area in admin.py file in the Django app.
from django.contrib import admin
from models import Student
admin.site.register(Student)
Creating Admin User and Password
To login into the Django admin panel, you need to create a username and password by using createsuperuser command. If you have already a username and password then you can skip this step.
python manage.py createsuperuser
Login Admin Panel
To login Django built-in admin panel you need to just start your Django development server by using the below command.
python manage.py runserver
visit this web address http://127.0.0.1:8000/admin/login/?next=/admin/ and enter the username and password that you have created using createsuperuser command.
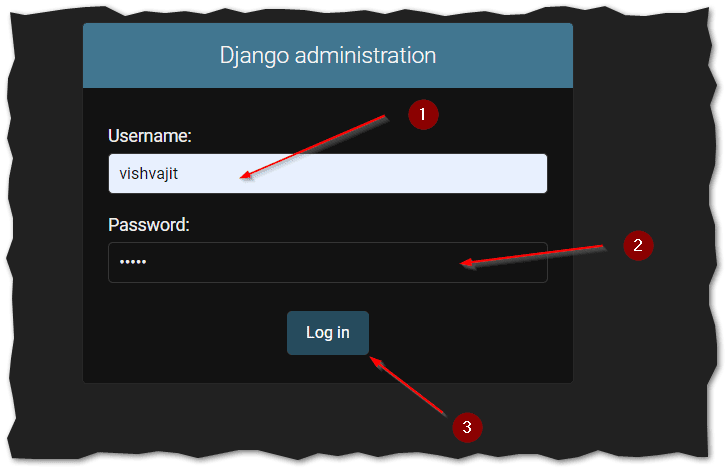
After login into the Django admin panel, you will see your new model and some other existing models provided by Django itself.
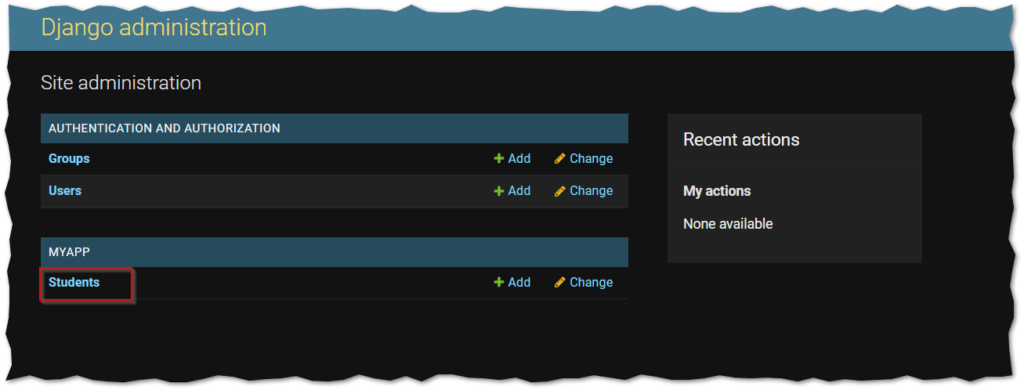
Add new Student
You can follow these steps to add a new student to the Student model.
- To add a new student, click on + Add button.

- Fill in all the details and click on save.

- After saving your details successfully, you will see the following window.
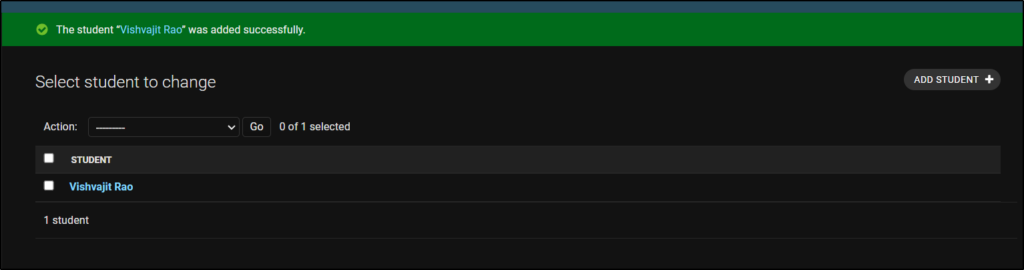
Retrieving Existing Object
To see the newly created object, click on that specific object. After clicking on that object, you will see the following window.
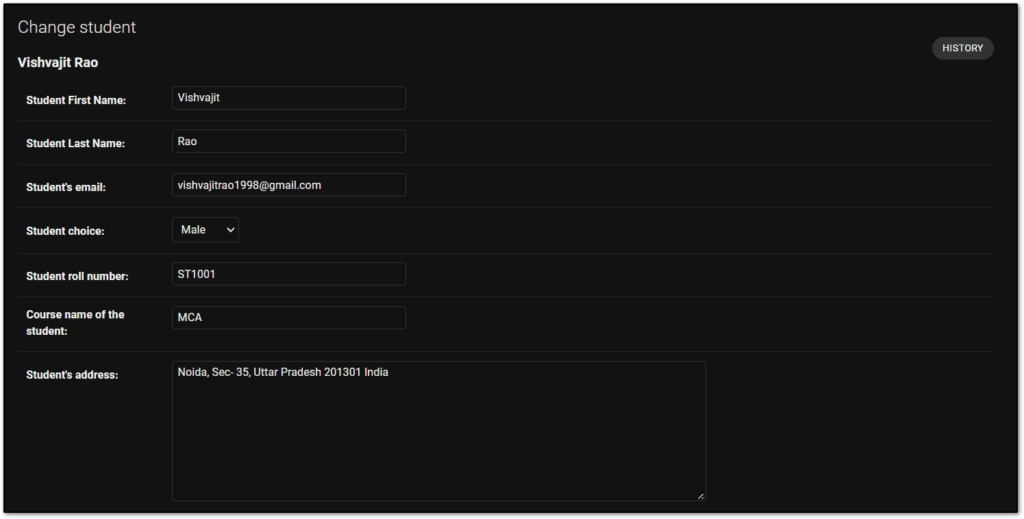
Update the object
To update the existing records you have to just click on that specific object fill the updated value and click save in the right bottom corner.

Deleting the object
To delete the object you can follow the below steps.
- Checkbox the particular object which you want to delete and choose to delete selected students from the above drop-down and click on Go.

- After that click on Yes, I’m sure.
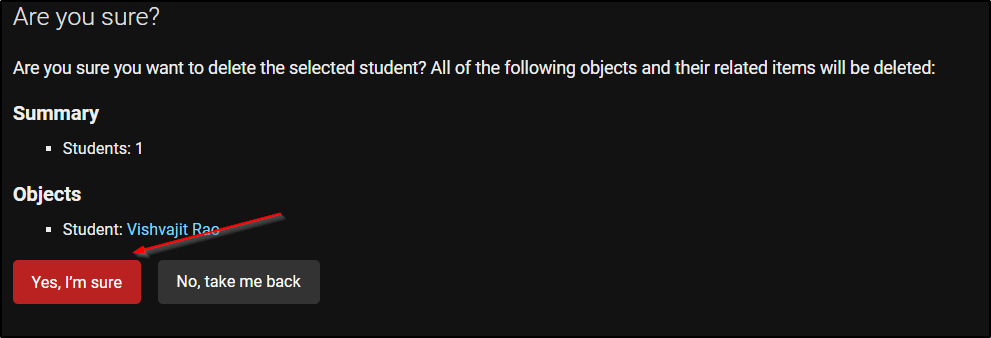
Conclusion
So throughout this article, we have seen a complete guide about what is Django model and how to use the Django model and create, delete and update the records.
In the previous tutorial, we have seen what is Django app and how to create a Django app in the Django application. Django models are basically a simple Python class that is a sub-class of Django.DB.models.Model class. Each Django class is mapped to a single database table.
In a later article, will see some advanced stuff about the Django model.
I hope after this article you don’t have any confusion regarding What is model in Django. If you like this article fill it out visit here and share this with known people who want to learn Django from zero to advanced.
Django related Articles
Thanks for reading….