Hi Django Developers, I hope you are doing good. In this guide, you will learn all about how to use Abstract model in Django and its use cases. Abstract classes in Django are one of the most important concepts, Especially when you want to put some common fields or information in other Django models ( Tables in Database ).
Before going deep dive into this article, I will tell some common Django important terms like What is Model in Django, What is inheritance, and also I will tell you about what is model inheritance in Django so that you can get more clarity with Django abstract model classes.
let’s discuss.
Headings of Contents
What are models in Django?
Models in Django are the most important components because it is defined as the source of information about our data. In other words In Django, models are simple Python classes inherited from django.db.models.Model
. Each Django model represents a single database table and each field of the Django model represents a column in a database table.
For example, I have created a Django model called Person
along with first_name, last_name, and age fields.
Example: Django Model
class People(models.Model):
first_name = models.CharField(max_length=40, db_column="first_name", verbose_name="First Name")
last_name = models.CharField(max_length=20, db_column="last_name", verbose_name="Last Name")
age = models.IntegerField(db_column="age")
def __str__(self):
return f"{self.first_name} {self.last_name}"
π Click Here to Learn all about Django Model
What is Inheritance in Python?
To understand the abstract model in Django, you should have knowledge about inheritance in Python because Django abstract model follows the inheritance concept.
Inheritance in Python is the process of inheriting or accessing the methods and properties from the other classes. There are multiple types of inheritance available in Python which are listed below.
- Single inheritance
- Multiple inheritances
- Multilevel inheritance
- Hierarchical inheritance
- Hybrid Inheritance
There are two types of classes available in Inheritance Parent or base class and child or derived class.
Parent class or Base Class:- Parent class is a class that being inherit from, it is also called a Parent class.
Child class or Derived Class:- A child class is a class that inherits from the other class. It is also called the derived class.
Let’s understand Python Inheritance using an example.
I have created two Django models Company and Employee where the Company is the Parent class and the Employee is the child class.
Example:- Inheritance in Python
class Company:
# constructor
def __init__(self, company_name):
self.company_name = company_name
# display company name
def display(self):
print(f"Company name is {self.company_name}")
class Employee(Company):
# constructor
def __init__(self, emp_name, company_name):
self.employee_name = emp_name
super().__init__(company_name)
# display employee name
def display_employee_name(self):
print(f"You name is {self.employee_name}")
# creating object of Employee class
emp1 = Employee("Vishvajit", "XYZ Company")
# access child class method
emp1.display_employee_name()
# access parent clss method
emp1.display()
Output
You name is Vishvajit
Company name is XYZ Company
As you can see in the above example, the Employee
class is inherited from the Company
class which means the Employee class has the authority to access all the methods and properties from Company ( Parent class ).
I hope you will understand what I mean to say.
π Types of inheritance in Python
What is model Inheritance in Django?
Model Inheritance in Django is almost the same as normal Python class Inheritance because the whole Django web framework is built from Python. Some basic points should be followed at the beginning of the page which means a base class should be a subclass of django.db.models.Model class.
The only decision, you have to make is whether you want to make a parent model with their own database table or just hold the common information which will only be visible through their child’s classes.
We can achieve model Inheritance in Django using three ways.
- Using Abstract base class.
- Using the Proxy model.
- Multi-table inheritance.
Throughout this article, we will cover only the abstract model, and the rest of the two will discuss in later tutorials.
What is an Abstract class in Django?
Abstract class in Django is the process of putting some common information that should be the same in other models. You can create Django abstract base class just by putting abstract=True in the Meta class in the Django model. The abstract base class will not create any table in the database it will just share the fields with other models who inherited from it.
Note:- Abstract class in Django is also called Django abstract model.
Example: Creating an abstract Model in Django
class College(models.Model):
college_name = models.CharField(max_length=100, db_column="college_name", verbose_name="College Name")
college_address = models.TextField(max_length=200, db_column="college_address", verbose_name="College Address")
def __str__(self):
return self.college_name
class Meta:
abstract = True
Why do you need an Abstract model in Django?
In real-life projects, we can have multiple Django models and some of those models can have common fields so instead of creating all common fields in each Django model we can create a Django abstract model along with all those common fields and inherit all the Django normal model from the abstract base class.
Let’s understand the Abstract base class through an example.
Example:
class Student(models.Model):
first_name = models.CharField("Student First Name:- ", max_length=30)
last_name = models.CharField("Student Last Name:- ", max_length=30)
full_name = models.CharField("Student Full Name:- ", max_length=40)
college_name = models.CharField(
max_length=100, db_column="college_name", verbose_name="College Name"
)
college_address = models.TextField(
max_length=200, db_column="college_address", verbose_name="College Address"
)
class CollegeEmployee(models.Model):
emp_first_name = models.CharField("Employee First Name:- ", max_length=30)
emp_last_name = models.CharField("Employee Last Name:- ", max_length=30)
college_name = models.CharField(
max_length=100, db_column="college_name", verbose_name="College Name"
)
college_address = models.TextField(
max_length=200, db_column="college_address", verbose_name="College Address"
)
As you can see in both above tables ( Student and CollegeEmployee ), some fields are the same like college_address and college_name. Suppose you are working on a real-life project and there are more than a hundred or thousand tables are exist then it will be very tedious to define the same fields again and again in all the Django models. So it is a better way to define a single Django abstract base class and define all the common fields inside, and inherit all the models from the abstract base class.
How to create an Abstract model in Django?
As we can discuss in the above abstract base class definition, the process of creating an abstract model in Django. First, you have to define a model which will be derived from the Model class which is defined inside django.db.models package class, and define all the common fields you want inside that, and after that define all the Django normal models which will be derived from an abstract base class.
models.py file: Creating Django Abstract Base class
from django.db import models
# Django abstract base class or Django abstract model
class College(models.Model):
college_name = models.CharField(
max_length=100, db_column="college_name", verbose_name="College Name"
)
college_address = models.TextField(
max_length=200, db_column="college_address", verbose_name="College Address"
)
class Meta:
abstract = True
# Django normal model inherited from Abstract class
class Student(College):
first_name = models.CharField("Student First Name:- ", max_length=30)
last_name = models.CharField("Student Last Name:- ", max_length=30)
full_name = models.CharField("Student Full Name:- ", max_length=40)
def __str__(self):
return "Student:- " + self.first_name + " College:- " + self.college_name
# Django normal model inherited from Abstract class
class CollegeEmployee(College):
emp_first_name = models.CharField("Employee First Name:- ", max_length=30)
emp_last_name = models.CharField("Employee last Name:- ", max_length=30)
emp_full_name = models.CharField("Employee last Name:- ", max_length=30)
def __str__(self):
return "Employee:- " + self.emp_first_name + " College:- " + self.college_name
admin.py file: Registering Django Model
from django.contrib import admin
from .models import Student, CollegeEmployee
admin.site.register(Student)
admin.site.register(CollegeEmployee)
After applying makemigrations and migrate command you can see your tables in the Django admin panel. Like below
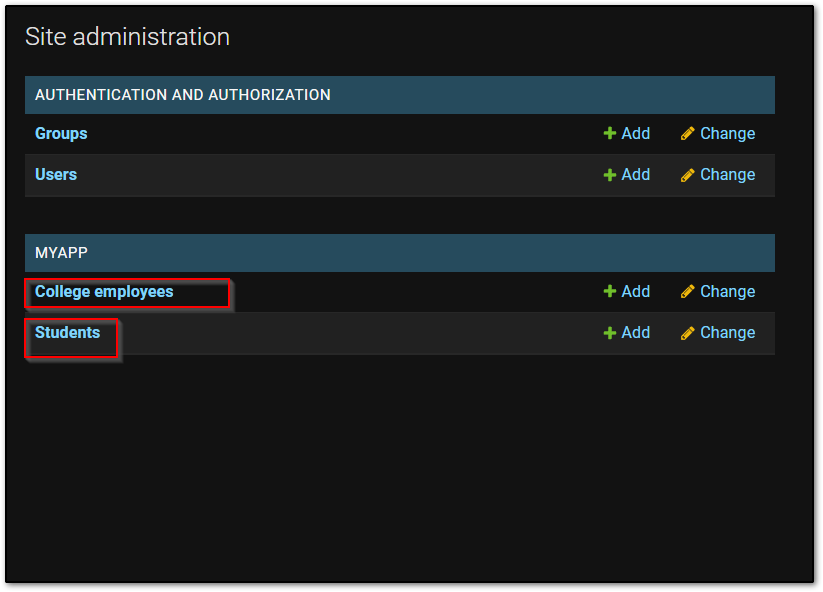
Once you will click on add icon to add a student or add a college employee, You will see, The two fields college_name and college_address will have been added. Whereas, We did not add these two fields ( college_name and college_address ) in each model separately, So that’s the power of the Django abstract model.
Note:- It might be, that you have been confused. Should the college name and college address same for all the students and college employees? the answer is No, It can differ according to students and employee information. As you can see below screenshot.


Conclusion
So, In this guide, we have covered all about How to use Abstract Model in Django with the help of the example. It is also called Django abstract class. The abstract model is one of the most popular and useful features in Django, To keep identical or common information for a number of Django models.
You should always use the Abstract model If you have a lot of Django models and they are sharing some common fields because it will save you a lot of effort and time.
I hope after reading this article, you don’t have any confusion regarding the abstract model in Django.
If you like this Django tutorial, please support and share this community.
Reference:- Django Abstract Model Docs
Happy Coding Journey π§βπ»π§βπ»
Thanks for your valuable timeβ¦. β€οΈβ€οΈππ