The date column is one of the most important columns in Pandas DataFrame specifically when you are going for time series analysis in Pandas DataFrame, In this article, we will see multiple ways to add date column in Pandas DataFrame with the help of examples.
Throughout this article, we are about to see how to add the current date or series of dates as a column into the Pandas DataFrame.
To add a date column in Pandas DataFrame, we must have a Pandas DataFrame, don’t worry I have already created a sample dataset for you as you can see below.

Let’s load this CSV dataset into Pandas DataFrame.
Here, I am using the Pandas read_csv() function in order to load CSV file data into Pandas DataFrame.To use the read_csv() function, first, we will have to import the read_csv() function from the Pandas library.
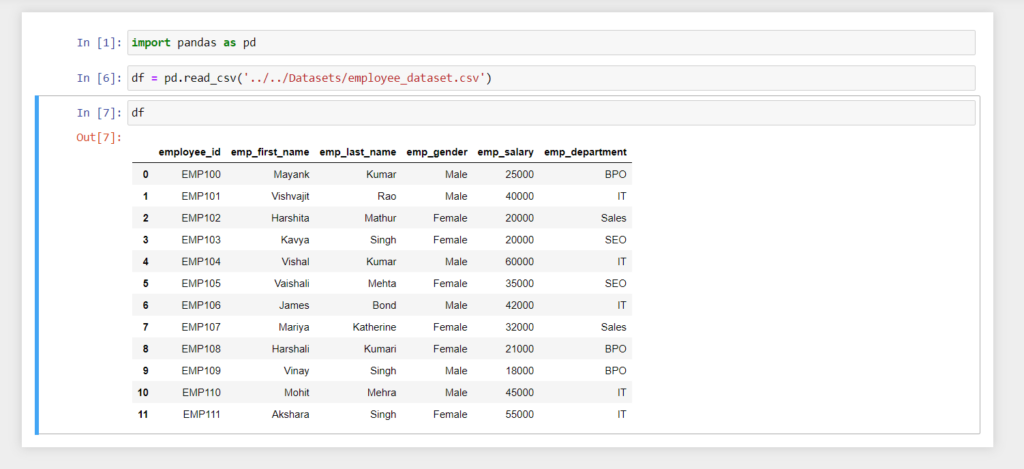
You can able to load CSV files into Pandas DataFrame in Multiple ways by reading our read CSV file into Pandas DataFrame tutorial.
As you can see in the above Pandas Sample DataFrame, There is no date column now we will add a date column in DataFrame.
Headings of Contents
How to add Date column in Pandas DataFrame
if I talk about the date column in Pandas DataFrame then it might be a current date or a series of dates but here we will learn how we can add a current date or series of dates as a column in Pandas DataFrame.
Let’s start,
Add Current Date in Pandas DataFrame
There are multiple ways to add a current date in the date column in Pandas DataFrame. In this section, we will add a column called joining_date which will store the current date as a value.
By Directly assigning the current date as a string:
This is the easiest way to add the current date column in Pandas DataFrame.To add a current date in the date column we will have to provide the current date as a string like ‘2023-12-01‘ to the new column name.
For example, In the above DataFrame, I have added the current date as you can see below.
import pandas as pd
df = pd.read_csv(
'../../Datasets/employee_dataset.csv'
)
df['joining_date'] = '2023-11-12'
print(df)

Code Explanation:
- Imported the Python pandas library as pd
- Load CSV dataset using read_csv() method into Pandas DataFrame.
- Assigned the current as a string into DataFrame’s new column called joining_date.
- Displayed the Pandas DataFrame.
Using Python datetime date.today() Method:
As know Python comes with a huge number of modules and packages, and datetime is one of the popular packages from them, there is no need to install DateTime because it comes with Python itself. To add a current date in the date column we need to import date class from datetiime module and then call the today() method of the date class.
In the below example, I have added the current date in the joining_date column using the Python datetime module.
import pandas as pd
from datetime import date
df = pd.read_csv(
'../../Datasets/employee_dataset.csv'
)
df['joining_date'] = date.today()
print(df)

Code Explanation:
- Imported the Python pandas library as pd
- Load CSV dataset using read_csv() method into Pandas DataFrame.
- Assigned the current date which is created from the date.today() into DataFrame’s new column called joining_date.
- Displayed the Pandas DataFrame.
Using Pandas pandas.Timestamp.today().date():
Pandas also has a class Timestamp which has a class method called today() that returns the current date time as an object and that object has a method called date() which returns the current date.
Let’s see how can we use pandas.Timestamp.today().date() to add a current date in the joining_date column.
import pandas as pd
df = pd.read_csv(
'../../Datasets/employee_dataset.csv'
)
df['joining_date'] = pd.Timestamp.today().date()
print(df)

Code Explanation:
- Imported the Python pandas library as pd
- Load CSV dataset using read_csv() method into Pandas DataFrame.
- Assigned the current date which is created from pd.Timestamp.today().date() into DataFrame’s new column called joining_date.
- Displayed the Pandas DataFrame.
Add a range of Dates Using the Pandas date_range() function
In all the above examples, we have added the current date in the joining_date column which are same for all the rows but sometimes our requirement might be to add a range of dates as a column In Pandas DataFrame.
date_range() function is one of the popular functions to return a fixed frequency date time range. Let’s generate a date range using the date_range() function and then add it to Pandas DataFrame.To import date_range(), it should be imported from the pandas package.
Let’s generate a range of dates and then add it to the Pandas DataFrame joining_date column.
import pandas as pd
df = pd.read_csv(
'../../Datasets/employee_dataset.csv'
)
date_column = pd.date_range(start='2023-11-01', periods=12, freq='D')
df['joining_date'] = date_column
print(df)
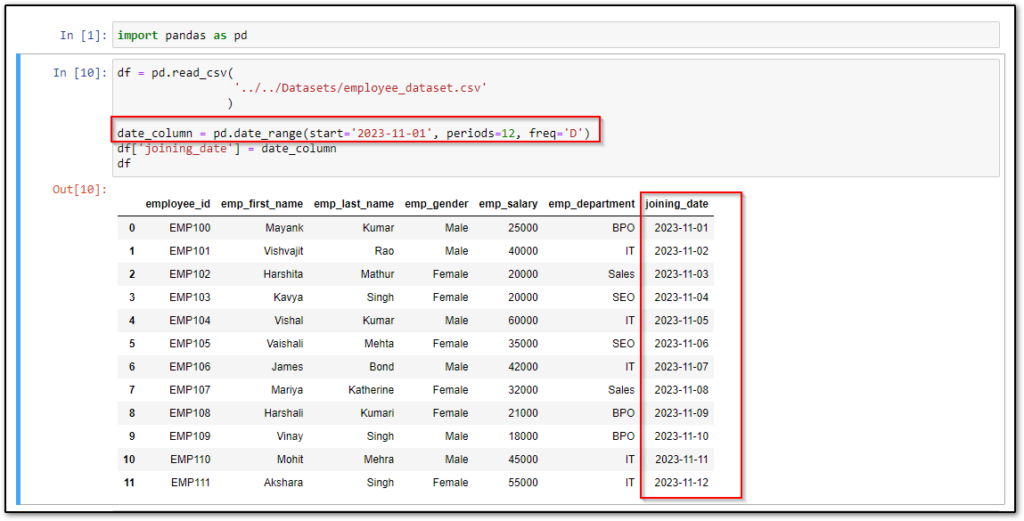
Code Explanation:
- Imported the Python pandas library as pd
- Load CSV dataset using read_csv() method into Pandas DataFrame.
- Created the date range using pandas date_range() function which is started from 1st November to 12th November because in DataFrame there are only 12 rows exist.
- Assigned the above-created date range into DataFrame’s new column called joining_date.
- Displayed the Pandas DataFrame.
Conclusion
Throughout this article, we have seen multiple ways to add date columns in Pandas DataFrame with the help of the examples. If your requirement is to add only the current date as a column in the Pandas DataFrame then the first three ways will be efficient for you and for the range of dates, you can go with the date_range() function which we have seen last examples.
If you found this article helpful, please share and keep visiting for further Pandas tutorials.
Thanks for your valuable time…..