Sometimes In Pandas DataFrame, a date column exists and we want to get day name from date in Pandas DataFrame to perform some operations on the name of the day or weekday, in that scenario First we will have to get the name of the weekday or day based on the data available in date column then we can perform operations on day name.
Let’s understand this with the help of a real-life example. Suppose you have a dataset of company employees that contains various fields of employees and date_of_joining is one field of them and now your requirement is to get all those Female employees whose date of joining is Friday but remember you don’t have a day name column in Pandas DataFrame then in that scenarios firstly you will have to get the column name based on the joining date available in date_of_joining column and then you can filter Female employees along with Friday weekday.
Let’s see this scenario through practice.
To get day name from the date in Pandas DataFrame, I have created a sample employees dataset in a CSV file along with various fields of employees, As you can see below.
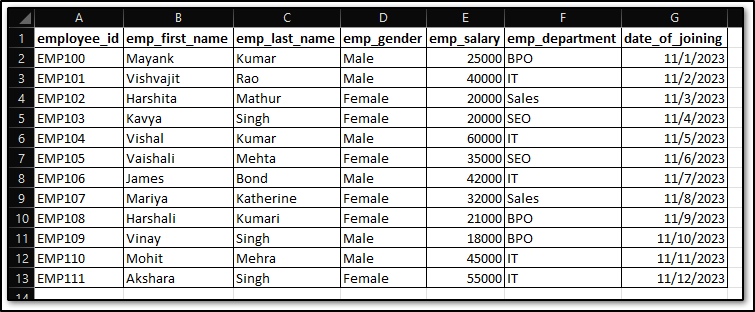
Now, I have loaded the above CSV dataset in Pandas DataFrame using the read_csv() method.
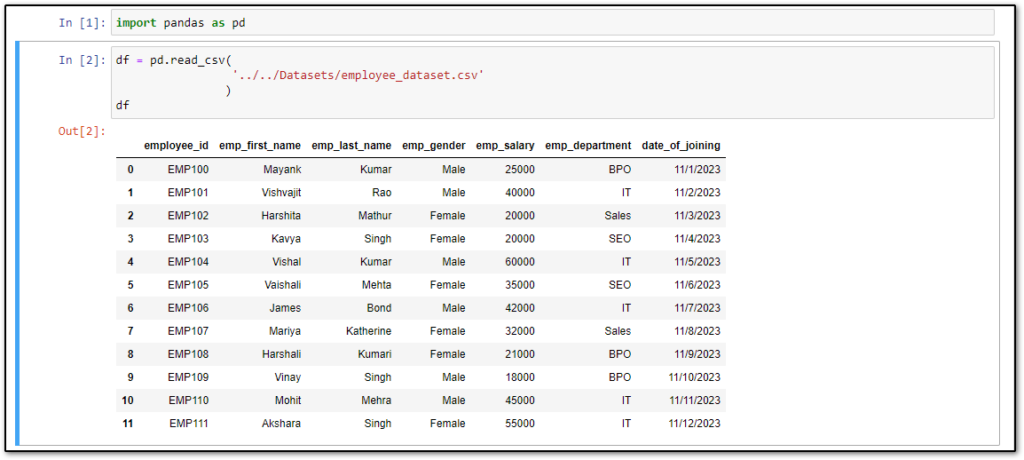
Note:- I am using Jupyter Notebook for writing code, You can go with any code editor as per your convenience.
If you don’t know how to read CSV files into Pandas DataFrame, Then 👉 click here to learn the complete article.
So far we have loaded the CSV file into Pandas DataFrame, now it’s time to get day name of the date in Pandas DataFrame.
But before applying any function, we will need to check the type of value available in the date_of_joining column of Pandas DataFrame.To check the data type of Pandas DataFrame column value use the below code.
import pandas as pd df = pd.read_csv( '../../Datasets/employee_dataset.csv' ) print(type(df['date_of_joining'][0]))
The output will be: str
You can see in below screenshot.

As you can see in the above output, the data type is str that’s why we cannot perform any function to get the day name. Firstly we need to convert the string date to the pandas Datetime object then we can get the day name from that object.
Let’s convert the string date to Pandas timestamp object by using the Pandas to_datetime() function.
Note:- If in your case, The Date type of date column in Pandas timestamp object or datetime object then you can ignore this convertion section.
Headings of Contents
Convert string date to date object in Pandas DataFrame
Pandas provides a function called to_datetime() which is used to convert string date time to Pandas Datetime object to perform operations on converted Pandas Datetime object.
to_datetime() function takes various parameters which you can explore by 👉 clicking here.
In the below Pandas code, I have converted the string date to the Pandas timestamp object.
import pandas as pd df = pd.read_csv( '../../Datasets/employee_dataset.csv' ) df['date_of_joining'] = pd.to_datetime(df['date_of_joining']) print(df)
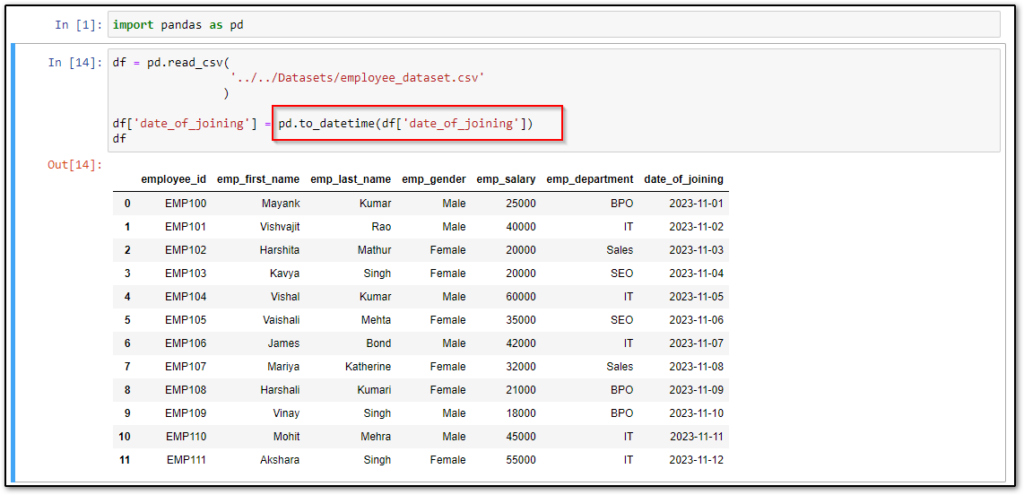
Now, Let’s check again the data type of value available in the date_of_joining column.

import pandas as pd df = pd.read_csv( '../../Datasets/employee_dataset.csv' ) df['date_of_joining'] = pd.to_datetime(df['date_of_joining']) type(df['date_of_joining'][0])
The Output will be:- pandas._libs.tslibs.timestamps.Timestamp
As you can see, I have successfully converted the string date time Pandas Timestamp object called Timestamp, now I am ready to perform any kind of date operations on top of the date_of_joining column.
Now let’s move to our main topic which was, to get the day name from the date column in Pandas DataFrame.
Get Day Name from Date in Pandas DataFrame
Pandas provides various ways to get the day name from the date in Pandas DataFrame and we will explore all the possible ways here. Here, I will add a new column called day_name which will store the day name from the corresponding date.
Let’s start.
Using day_name() function
day_name() is a method that is written in the Pandas DateTimeIndex class and this method is responsible for returning the name of the day for the corresponding Pandas TimeStamp object.
In the below example, First I converted the string date to Pandas TimeStamp object, and then I implemented the day_name() method to get the day name.
import pandas as pd df = pd.read_csv( '../../Datasets/employee_dataset.csv' ) df['date_of_joining'] = pd.to_datetime(df['date_of_joining']) df['day_name'] = df['date_of_joining'].dt.day_name() print(df)
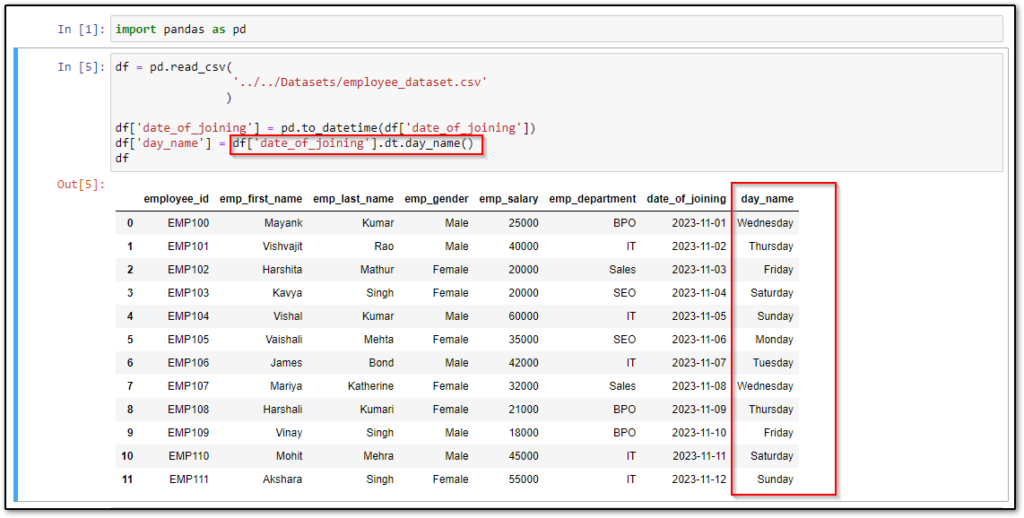
Code Explanation
- Imported the pandas as pd
- Loaded the CSV file into Pandas DataFrame using the Pandas read_csv() method.
- Converted the string date to Pandas date object using Pandas to_datetime() method.
- Get the name of the day from the date using the day_name() method and store it in the day_name column.
- Displayed the Pandas DataFrame.
Using dayofweek property
dayofweek is another property that can be used to get the day name from Pandas TimeStamp object but this is a little different from the day_name() method, instead of returning the day name it returns the day of the week in integer format like 0 for Monday, 1 for Tuesday and 2 for Wednesday and so on.
To get the proper day name from the integer value we have to map that integer value to the corresponding day name using 👉 Python Dictionary and then we can implement Pandas apply() function to get the day name.
Let’s see how can we do that.
import pandas as pd df = pd.read_csv( '../../Datasets/employee_dataset.csv' ) df['date_of_joining'] = pd.to_datetime(df['date_of_joining']) days = {0:'Monday', 1:'Tuesday', 2:'Wednesday', 3:'Thursday', 4:'Friday', 5:'Saturday', 6:'Sunday'} df['dayofweek'] = df['date_of_joining'].dt.dayofweek df['day_name'] = df['dayofweek'].apply(lambda x: days[x]) print(df)
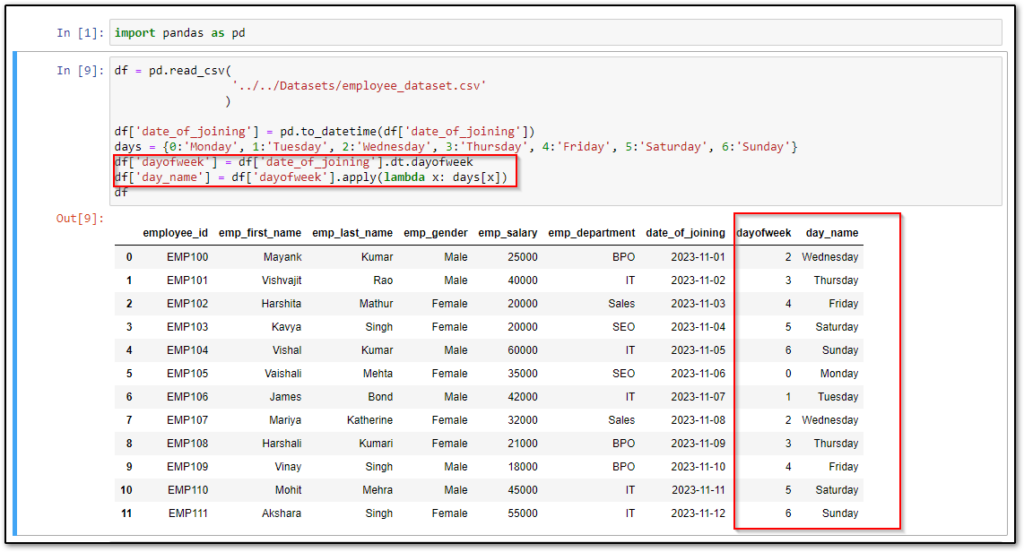
Code Explanation
- Imported the pandas as pd
- Loaded the CSV file into Pandas DataFrame using the Pandas read_csv() method.
- Converted the string date to Pandas date object using Pandas to_datetime() method.
- Defined a Python Dictionary that stored the day number as key and day name or weekday as value.
- Created a new column dayofweek in Pandas DataFrame that stored the day number returns from dayofweek property.
- Applied the DataFrame apply() function on top of dayofweek column to get the day name and stored it in the day_name column.
- Displayed the Pandas DataFrame.
This is how we can get Day name from date column in Pandas DataFrame.Now let’s see some problem-based questions that might be asked in data analysis interviews.
Problem-Based Questions
These questions might be asked in Data analysis or Data engineering interviews.
Problem 1:- Get all the employees whose joining day is Friday:
Ans:- Suppose, In the dataset, joining_date or any other date column exists.
To solve this question, Firstly you need to get the day name from the date object (After converting the date string to the pandas Timestamp object ) and after that, you will need to filter all those employees whose day of joining is Friday.
Let’s see through practical how can we solve this kind of question.
import pandas as pd df = pd.read_csv( '../../Datasets/employee_dataset.csv' ) df['date_of_joining'] = pd.to_datetime(df['date_of_joining']) df['day_name'] = df['date_of_joining'].dt.day_name() new_df = df.loc[df['day_name'] == 'Friday', df.columns] print(new_df)

Code Explanation
- Imported the pandas as pd
- Loaded the CSV file into Pandas DataFrame using the Pandas read_csv() method.
- Converted the string date to Pandas date object using Pandas to_datetime() method.
- Getting the day name from the date object and storing it in the day_name column.
- Used the Pandas loc property to get a group of rows and columns by labels and filter day_name with Friday value and used df.columns to get all the columns in the result.
Problem 1:- Get all the employees whose joining day is Friday and Gender is Female:
In the above example, we had one condition that was the joining day should be Friday but we want to all those Female employees whose day of joining is Friday.
The process will be the same as the above code. The only difference is that we need to define two conditions instead of one as you can see below.
This time I didn’t use loc property.
import pandas as pd df = pd.read_csv( '../../Datasets/employee_dataset.csv' ) df['date_of_joining'] = pd.to_datetime(df['date_of_joining']) df['day_name'] = df['date_of_joining'].dt.day_name() new_df = df[(df['day_name'] == 'Saturday') & (df['emp_gender'] == 'Female')] print(new_df)

Same as you can make multiple conditions based on the day name or weekday and date_of_joining column.
Let’s wrap up this article here, I hope you don’t have any confusion regarding how to get the day name from date column in Pandas DataFrame.
Explore Useful Pandas Articles:
- How To Add a Column in Pandas Dataframe
- How to Replace Column Values in Pandas DataFrame
- How to Convert Excel to JSON in Python
- How to convert DataFrame to HTML in Python
- How to Delete a Column in Pandas DataFrame
- How to convert SQL Query Result to Pandas DataFrame
- How to Convert Dictionary to Excel in Python
- How to Convert Excel to Dictionary in Python
- How to Rename Column Name in Pandas DataFrame
Conclusion
In today, article we have seen all about how to get day name from date in Pandas DataFrame with the help of the day_name() method and dayofweek property. you can use any one of them but I will recommend that you, always go with the day_name() method because it is more convenient in comparison to dayofweek property.
If you found this article helpful, please share and keep visiting for further Pandas articles.
Happy Coding! 🙏🙏