In this article we are going to see how to get top 10 highest values in Pandas DataFrame with the help of the examples so that you can get
get the top 10 highest values easily.
Pandas DataFrame has a method called nlargest() that is used to access the n largest values where n represents the number of rows that we want to access.
To get the 10 highest values we must have the Pandas DataFrame, Now let me create Pandas DataFrame where we will see the process getting the 10 highest values with the help of the nlargest() method.
Headings of Contents
Sample Pandas DataFrame
I have prepared a sample CSV file along with some records as you can see below.
employees.csv |
---|
emp_full_name,emp_email,emp_gender,emp_salary,emp_department,date_of_joining Mayank Kumar,[email protected],Male,25000,BPO,11/1/2023 Vishvajit Rao,[email protected],Male,40000,IT,11/2/2023 Harshita Mathur,[email protected],Female,20000,Sales,11/3/2023 Kavya Singh,[email protected],Female,20000,SEO,11/4/2023 Vishal Kumar,[email protected],Male,60000,IT,11/5/2023 Vaishali Mehta,[email protected],Female,35000,SEO,11/6/2023 Vaishali Mehta,[email protected],Female,35000,SEO,11/6/2023 James Bond,[email protected],Male,42000,IT,11/7/2023 Mariya Katherine,[email protected],Female,32000,Sales,11/8/2023 Mariya Katherine,[email protected],Female,40000,Sales,11/8/2023 Harshali Kumari,[email protected],Female,21000,BPO,11/9/2023 Vinay Singh,[email protected],Male,18000,BPO,11/10/2023 Vinay Mehra,[email protected],Male,45000,IT,11/11/2023 Akshara Singh,[email protected],Female,55000,IT,11/12/2023 |
Now, I have created a Pandas DataFrame with the help of the read_csv() method.
import pandas as pd df = pd.read_csv( '../../Datasets/employees.csv' ) print(df)
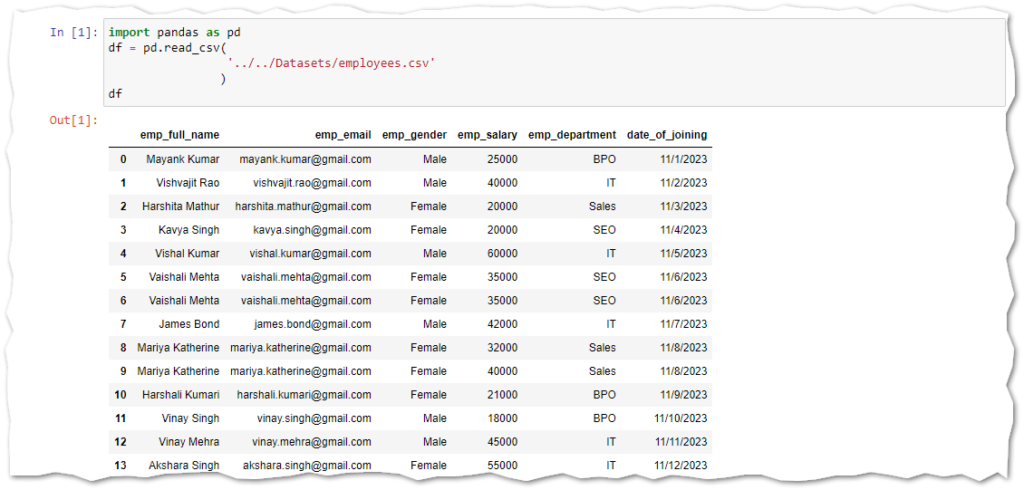
Now, Let’s see how we can get top 10 highest values in the Pandas DataFrame with the help of the nlargest() method.
Get Top 10 Highest Values in Pandas DataFrame
Before using nlargest() method let’s see a little about nlargest() and its parameters.
nlargest() is a Pandas DataFrame method that is used to return the first n rows order by column in descending order.
It takes some parameters which are listed below.
- n:- It indicates the number of rows we want to access.
- columns:- Column names to order by.
- keep:- It takes three values {‘first’, ‘last’, ‘all’} default is first.
- first: prioritize the first occurrence(s)
- last: prioritize the last occurrence(s)
- all: keep all the ties of the smallest item even if it means selecting more than n items.
Now, we can use nlargest() method to get top 10 highest values in Pandas DataFrame.
Note:- If you will use nlargest() without n and columns parameter then you will get an error. Note:- You can pass column names inside the Python list to the columns parameter of the columns parameter.
Example: Top 10 Highest Rows By Employee Salary
import pandas as pd df = pd.read_csv( '../../Datasets/employees.csv' ) new_df = df.nlargest(10, columns='emp_salary') print(new_df)
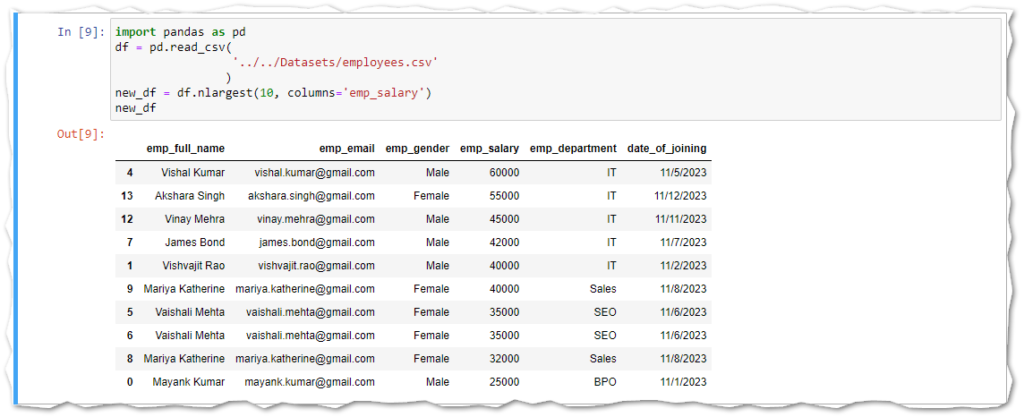
Note:- In the above example, keep the parameter by default using the 'first' value which means it will get only the first occurrence.
Let’s see both another value ‘last‘ and ‘all‘ of the keep parameter.
Example:
import pandas as pd df = pd.read_csv( '../../Datasets/employees.csv' ) new_df = df.nlargest(10, columns='emp_salary', keep='last') new_df
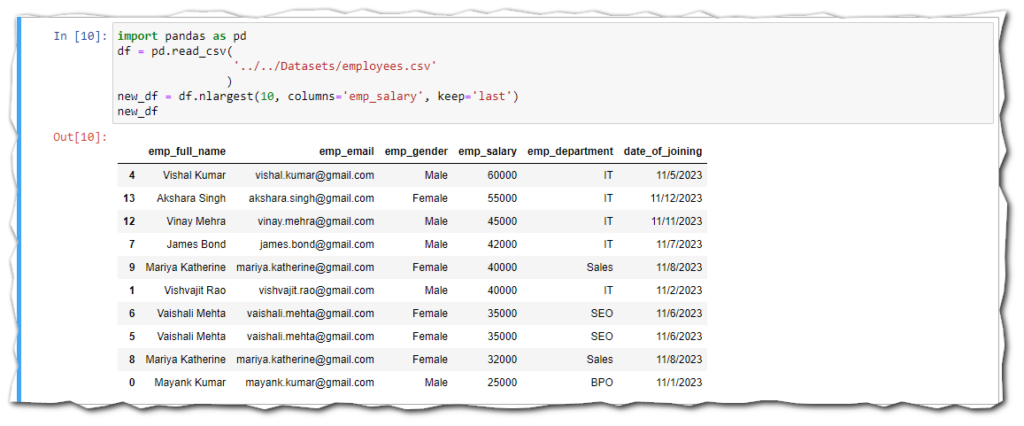
Note:- 'last' value inside the keep parameter prioritizes the last occurrence of the items.
Example
import pandas as pd df = pd.read_csv( '../../Datasets/employees.csv' ) new_df = df.nlargest(10, columns='emp_salary', keep='all') print(new_df)

Note:- When you are using keep='all', The number of elements can kept beyond the n number if there are duplicate values for the smallest elements.
This is how you can use nlargest() method to get the 10 highest values in Pandas DataFrame.
Helpful Pandas Articles
- How to convert Dictionary to CSV
- How to convert YML to Dictionary
- How to convert Excel to Dictionary
- How to Convert String to DateTime in Python
- How to Sort the List Of Dictionaries By Value in Python
- How To Add a Column in Pandas Dataframe
- How to Replace Column Values in Pandas DataFrame
- How to Convert Excel to JSON in Python
- How to Drop Duplicate Rows in Pandas DataFrame
- How to use GroupBy in Pandas DataFrame
- How to convert DataFrame to HTML in Python
- How to Delete a Column in Pandas DataFrame
- How to convert SQL Query Result to Pandas DataFrame
- How to Convert Dictionary to Excel in Python
- How to Convert Excel to Dictionary in Python
- How to Rename Column Name in Pandas DataFrame
- How to Get Day Name from Date in Pandas DataFrame
- How to Split String in Pandas DataFrame Column
- How to Display First 10 Rows in Pandas DataFrame
Conclusion
nlargest() method is the Pandas DataFrame method that will apply only on Pandas DataFrame especially used when you want to get some specific number of highest value from Pandas DataFrame.
This is important Pandas DataFrame method an interview and Pandad applications point of view. If you found this article helpful, please share and keep visiting for further Pandas tutorials.
Thanks for reading this ….