In this pandas tutorial, we are going to see how to get top 10 lowest values in Pandas DataFrame with the help of the examples so that you can get
get the top 10 lowest values easily.
Pandas DataFrame has a method called nsmallest() that is used to access the n largest values where n represents the number of rows that we want to access.
To get the 10 minimum values we must have the Pandas DataFrame, Now let me create Pandas DataFrame where we will see the process of getting the 10 lowest values with the help of the nsmallest() method.
Headings of Contents
Sample Pandas DataFrame
I have prepared a sample CSV file along with some records as you can see below.
employees.csv |
---|
emp_full_name,emp_email,emp_gender,emp_salary,emp_department,date_of_joining,age Mayank Kumar,[email protected],Male,25000,BPO,11/1/2023,25 Vishvajit Rao,[email protected],Male,40000,IT,11/2/2023,30 Harshita Mathur,[email protected],Female,20000,Sales,11/3/2023,23 Kavya Singh,[email protected],Female,20000,SEO,11/4/2023,24 Vishal Kumar,[email protected],Male,60000,IT,11/5/2023,28 Vaishali Mehta,[email protected],Female,35000,SEO,11/6/2023,27 Vaishali Mehta,[email protected],Female,35000,SEO,11/6/2023,25 James Bond,[email protected],Male,42000,IT,11/7/2023,23 Mariya Katherine,[email protected],Female,32000,Sales,11/8/2023,29 Mariya Katherine,[email protected],Female,40000,Sales,11/8/2023,31 Harshali Kumari,[email protected],Female,21000,BPO,11/9/2023,20 Vinay Singh,[email protected],Male,18000,BPO,11/10/2023,24 Vinay Mehra,[email protected],Male,45000,IT,11/11/2023,33 Akshara Singh,[email protected],Female,55000,IT,11/12/2023,30 |
Now, I have loaded the above CV file into Pandas DataFame with the help of the read_csv() method.
import pandas as pd df = pd.read_csv( '../../Datasets/employees.csv' ) print(df)
After loading the CSV into DataFrame, The DataFrame will look like this.
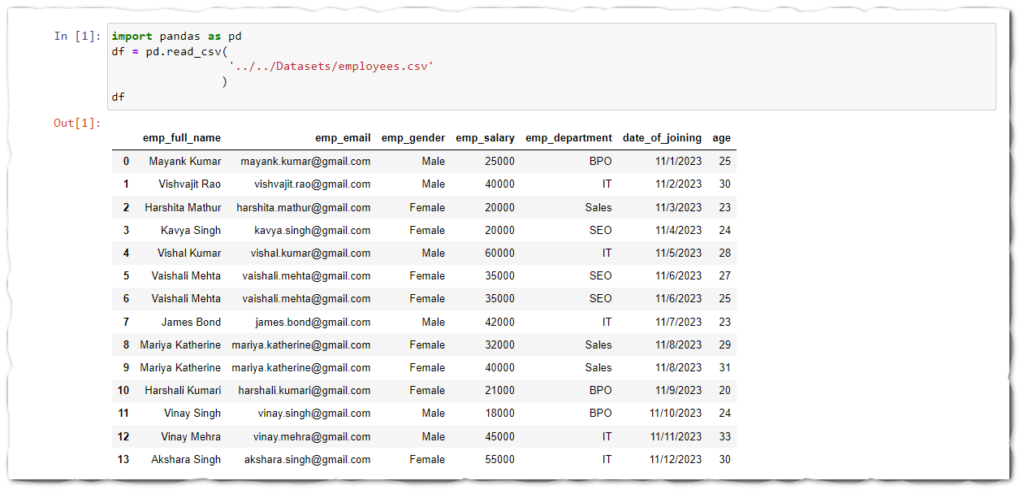
Now, Let’s see how we can get top 10 lowest values in the Pandas DataFrame with the help of the nsmallest() method.
Get Top 10 Lowest Values in Pandas DataFrame
Before using the nsmallest() method let’s see a little about it and its parameters.
nsmallest() is a Pandas DataFrame method that is used to return the first n rows order by column in ascending order. It takes some parameters which are listed below.
- n:- It indicates the number of rows we want to access. It should be an integer value.
- columns:- Column names to order by.
- keep:- It takes three values {‘first’, ‘last’, ‘all’} default values are first.
- first : prioritize the first occurrence(s)
- last : prioritize the last occurrence(s)
- all : keep all the ties of the smallest item even if it means selecting more than n items.
Now, We can use the nsmallest() method to get the top 10 minimum values in Pandas DataFrame.
Note:- If you will use nsmallest() without n and columns parameter then you will get an error. Note:- You can pass column names inside the Python list to the columns parameter of the columns parameter.
Example: Top 10 Lowest Rows By Employee Salary in Pandas DataFrame
import pandas as pd df = pd.read_csv( '../../Datasets/employees.csv' ) new_df = df.nsmallest(10, columns='emp_salary') print(new_df)
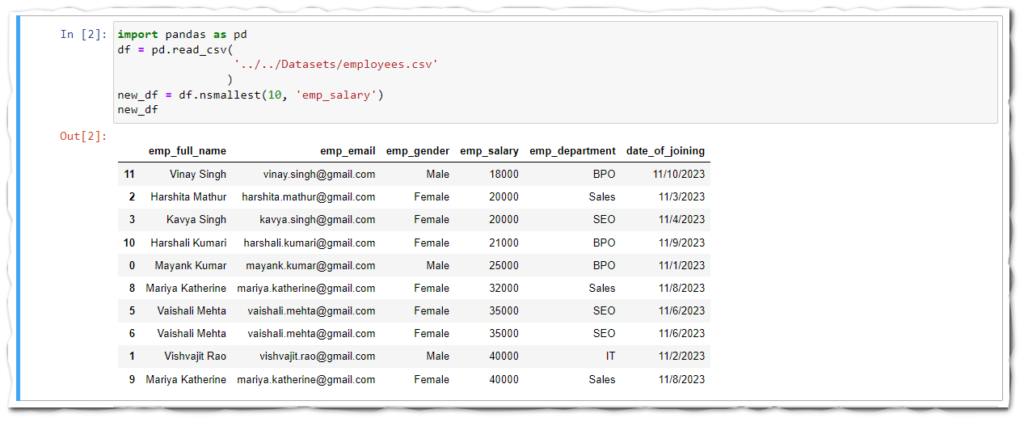
Note:- In the above example, keep parameter by default using the 'first' value which means it will get only first occurrence of values.
Let’s see the ‘last‘ and ‘all‘ values of the keep parameter one by one with the help of the examples.
Example:- Using the last value in the keep parameter of the nsmallest()
import pandas as pd df = pd.read_csv( '../../Datasets/employees.csv' ) new_df = df.nsmallest(10, columns='emp_salary', keep='last') print(new_df)
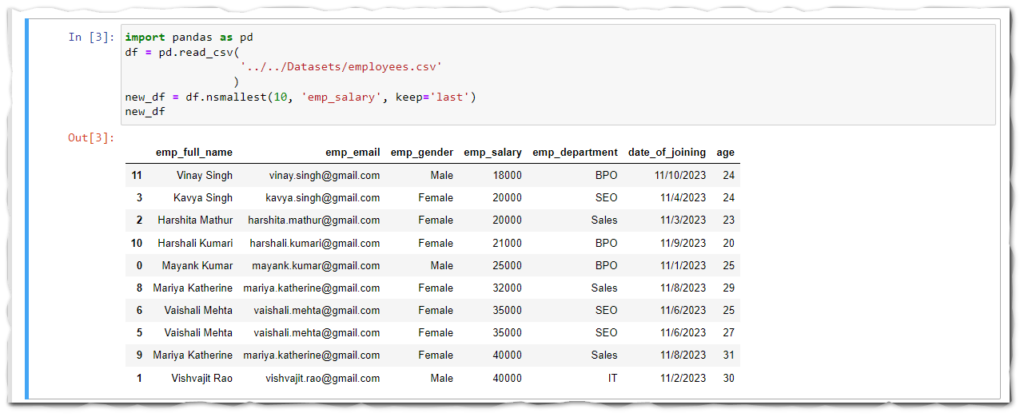
Note:- 'last' value inside the keep parameter, always prioritize the last occurrence of the items or values.
Example:- Using all values in keep parameter of the nsmallest()
import pandas as pd df = pd.read_csv( '../../Datasets/employees.csv' ) new_df = df.nsmallest(10, columns='emp_salary', keep='all') print(new_df)
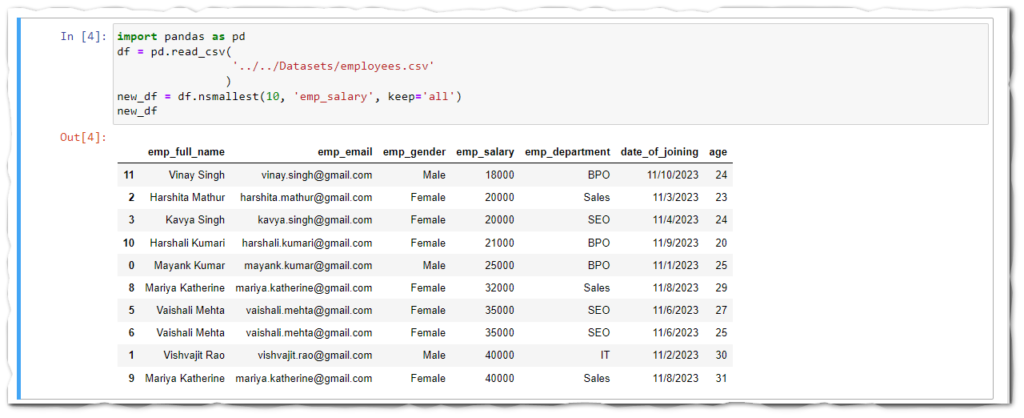
Note:- When you are using keep='all', The number of elements can kept beyond the n number if there are duplicates values for smallest elements.
Get 10 Lowest Values in Pandas DataFrame based on Multiple Columns
So far we have seen how we can get to the lowest or minimum value in Pandas DataFrame based on a single column name and now we will how we can use multiple column names in the columns parameter of the nsmallest() method.
Pass all the column names as a list into the columns parameter of the nsmallest() method.
In my case, I am going to pass emp_salary and age as a Python list into the columns parameter to get top 10 lowest values in Pandas DataFrame.
import pandas as pd df = pd.read_csv( '../../Datasets/employees.csv' ) new_df = df.nsmallest(10, columns=['emp_salary', 'age']) print(new_df)

This is how you can use nsmallest() method to get the 10 lowest values in Pandas DataFrame.
Pandas Useful Articles
- How to convert DataFrame to HTML in Python
- How to Delete a Column in Pandas DataFrame
- How to convert SQL Query Result to Pandas DataFrame
- How to Convert Dictionary to Excel in Python
- How to Convert Excel to Dictionary in Python
- How to Rename Column Name in Pandas DataFrame
- How to Get Day Name from Date in Pandas DataFrame
- How to Split String in Pandas DataFrame Column
- How to Display First 10 Rows in Pandas DataFrame
- How to convert Dictionary to CSV
- How to convert YML to Dictionary
- How to convert Excel to Dictionary
- How to Convert String to DateTime in Python
- How to Sort the List Of Dictionaries By Value in Python
- How To Add a Column in Pandas Dataframe
- How to Replace Column Values in Pandas DataFrame
- How to Convert Excel to JSON in Python
- How to Drop Duplicate Rows in Pandas DataFrame
- How to use GroupBy in Pandas DataFrame
- How to Get Top 10 Highest Values in Pandas DataFrame
Conclusion
smallest() method is the Pandas DataFrame method that will apply only on Pandas DataFrame especially used when you want to get some specific number of lowest value from Pandas DataFrame.
This is an important Pandas DataFrame method for important points of view and for Pandas Applications.
Thanks for reading this ….