In this article, we will see How to explode multiple columns in Pandas with the help of the example. The explode() method is the Pandas DataFrame method that is used to transform each element of a list into rows.
Throughout this article, we explore all Panda’s explode multiple columns with the help of different examples so that you can easily any question regarding the Python explode() function.
Before using explode(), we must know the Pandas explode() method and its parameters.
Let’s see.
Headings of Contents
Pandas explode() Method
It is a Pandas DataFrame method that is always used to transform each element of the list into rows and also replace index values.
explode() method returns a new DataFrame.
Parameters:
Pandas explode() method accepts two arguments:
- column:- Colume to be explode. It can be a single or a list of columns.
- ignore_index:- If it is True, the resulting index will be 0, 1, 2,…n-1
Let’s see some examples of Pandas explode list to row.
Explode multiple columns in Pandas
To apply the Python Pandas explode() method we must have a Python DataFrame, let’s create a sample Pandas DataFrame.here I am using the Jupyter Notebook tool, You can use any tool at your convenience.
Use the below code to create a sample Pandas DataFrame.

from pandas import DataFrame dictionary = [ { "name": "Vishvajit", "gender": "Male", "age": 25, "skills": ["Python", "SQL", "AWS"] }, { "name": "Vinay", "gender": "Male", "age": 20, "skills": ["Finance", "Accounting", "Excel"] }, { "name": "Harshita", "gender": "Female", "age": 24, "skills": ["Graphics Design", "Video Editing"] } ] df = DataFrame(data=dictionary) print(df)
After executing the above code, The sample DataFrame will look like this.
name gender age skills
0 Vishvajit Male 25 [Python, SQL, AWS]
1 Vinay Male 20 [Finance, Accounting, Excel]
2 Harshita Female 24 [Graphics Design, Video Editing]
Now the requirement is to transform each item of the list in column skills into a separate row.
Let’s see how can we do that.
Example: Pandas explode list to rows
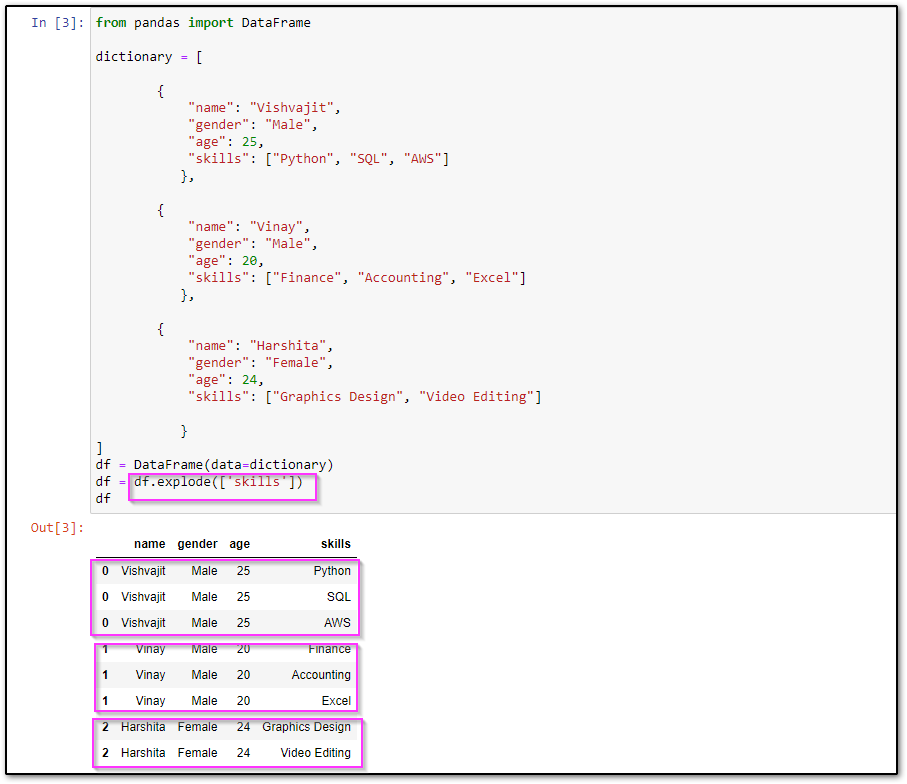
from pandas import DataFrame dictionary = [ { "name": "Vishvajit", "gender": "Male", "age": 25, "skills": ["Python", "SQL", "AWS"] }, { "name": "Vinay", "gender": "Male", "age": 20, "skills": ["Finance", "Accounting", "Excel"] }, { "name": "Harshita", "gender": "Female", "age": 24, "skills": ["Graphics Design", "Video Editing"] } ] df = DataFrame(data=dictionary) df = df.explode(['skills']) print(df)
After applying the Pandas explode() method the output will be.
name gender age skills
0 Vishvajit Male 25 Python
0 Vishvajit Male 25 SQL
0 Vishvajit Male 25 AWS
1 Vinay Male 20 Finance
1 Vinay Male 20 Accounting
1 Vinay Male 20 Excel
2 Harshita Female 24 Graphics Design
2 Harshita Female 24 Video Editing
As you can see in the above output, the index number is the same for each item of the list for a particular employee but sometimes we want to unique index number for each row then we can use the second parameter of the explode() method which is ignore_index.
To define a unique index for each row, we have to pass True to ignore_index parameter.
Example: Explode a column in Pandas
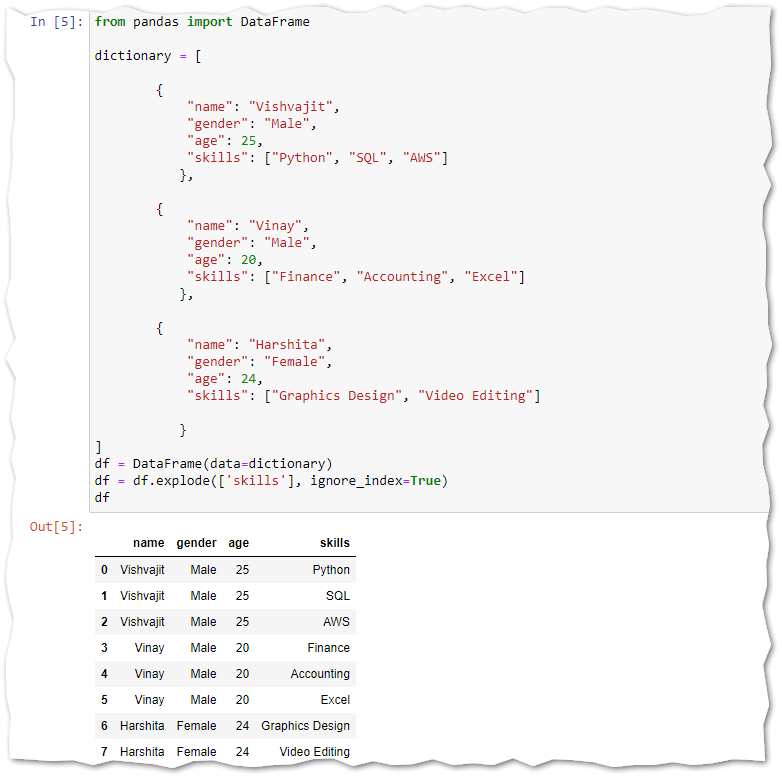
from pandas import DataFrame dictionary = [ { "name": "Vishvajit", "gender": "Male", "age": 25, "skills": ["Python", "SQL", "AWS"] }, { "name": "Vinay", "gender": "Male", "age": 20, "skills": ["Finance", "Accounting", "Excel"] }, { "name": "Harshita", "gender": "Female", "age": 24, "skills": ["Graphics Design", "Video Editing"] } ] df = DataFrame(data=dictionary) df = df.explode(['skills'], ignore_index=True) print(df)
The Output of the above code will be like this.
name gender age skills
0 Vishvajit Male 25 Python
1 Vishvajit Male 25 SQL
2 Vishvajit Male 25 AWS
3 Vinay Male 20 Finance
4 Vinay Male 20 Accounting
5 Vinay Male 20 Excel
6 Harshita Female 24 Graphics Design
7 Harshita Female 24 Video Editing
In all the above examples, we have seen the Pandas explode() method to explode the single column but sometimes we need to explode the multiple columns.
To explode multiple columns in Pandas, pass the column names as a list to the first parameter of the explode() method.
Note:- Length of the list must be the same in all the columns.
Let’s see an example.
Example: Explode multiple columns in Pandas

from pandas import DataFrame dictionary = [ { "name": "Vishvajit", "gender": "Male", "age": 25, "skills": ["Python", "SQL"], "course": ["BCA", "MCA"] }, { "name": "Vinay", "gender": "Male", "age": 20, "skills": ["Accounting", "Excel"], "course": ["BCOM", "MCOM"] }, { "name": "Harshita", "gender": "Female", "age": 24, "skills": ["Graphics Design", "Video Editing"], "course": ["BCA", "MCA"] } ] df = DataFrame(data=dictionary) df = df.explode(['skills', 'course'], ignore_index=True) df
After exploring the multiple columns the output will be:
name gender age skills course
0 Vishvajit Male 25 Python BCA
1 Vishvajit Male 25 SQL MCA
2 Vinay Male 20 Accounting BCOM
3 Vinay Male 20 Excel MCOM
4 Harshita Female 24 Graphics Design BCA
5 Harshita Female 24 Video Editing MCA
Helpful Pandas Articles
- How to convert Dictionary to CSV
- How to convert YML to Dictionary
- How to convert Excel to Dictionary
- How to Convert String to DateTime in Python
- How to Sort the List Of Dictionaries By Value in Python
- How To Add a Column in Pandas Dataframe
- How to Replace Column Values in Pandas DataFrame
- How to Convert Excel to JSON in Python
- How to Drop Duplicate Rows in Pandas DataFrame
- How to use GroupBy in Pandas DataFrame
- How to convert DataFrame to HTML in Python
- How to Delete a Column in Pandas DataFrame
- How to convert SQL Query Result to Pandas DataFrame
- How to Convert Dictionary to Excel in Python
- How to Convert Excel to Dictionary in Python
- How to Rename Column Name in Pandas DataFrame
- How to Get Day Name from Date in Pandas DataFrame
- How to Split String in Pandas DataFrame Column
Conclusion
Throughout this article, we have seen the Pandas explode() method to explode the single and multiple columns with the examples. There is a high chance in the coding interview, This question might be asked by the interviewer if you are going for a data engineering, or data analyst job profile or even for a Python developer job profile.
If you found this article helpful, please share and keep visiting for further pandas tutorials.