In this Pandas tutorial, you will see how to delete column from Pandas DataFrame, Columns can be single or multiple. This is the most common requirement in Pandas-based applications where most people get confused about which method is used to delete the column in Pandas DataFrame but don’t worry I will explain to you more than one way in order to delete one or single column in Pandas DataFrame with the help of examples.
To drop the column Pandas DataFrame, firstly we must have a Pandas DataFrame. Here I have created a simple Pandas DataFrame as you can see below.
👉 Explore more about Pandas DataFrame:- Click Here
from pandas import DataFrame
dictionary = {
"name": ["Vishvajit", "Manish", "Ankit", "Harshita", "Manisha", "Aysha"],
"age": [25, 26, 21, 24, 23, 22],
"gender": ['Male', 'Male', 'Male', 'Female', 'Female', 'Female'],
"country": ['India', 'Australia', 'India', 'US', 'UK', 'India']
}
df = DataFrame(data=dictionary)
print(df)
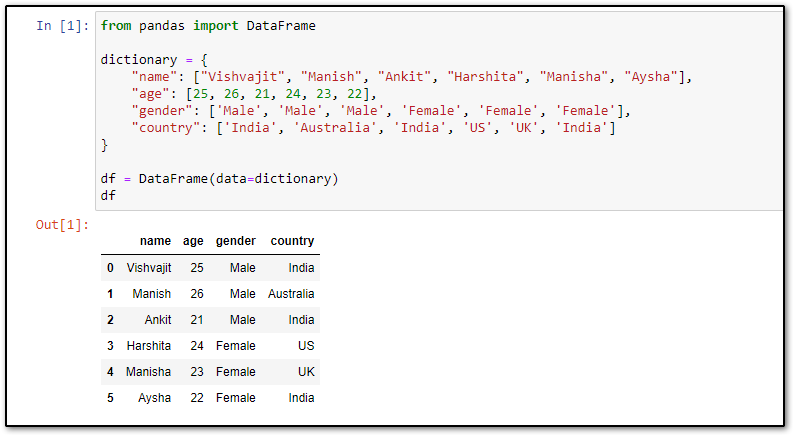
We have successfully created the Pandas DataFrame as you can see in the picture, now it’s time to see methods to remove columns in Pandas DataFrame.
Headings of Contents
Pandas DataFrame drop() Method
drop() is a method that is always used on top of Pandas DataFrame.It takes multiple parameters. The Pandas DataFrame drop() method is used to delete specified labels from rows and columns. The drop() method removes rows or columns by specifying label names and corresponding axes or directly specifying column or index names.
Syntax:
DataFrame.drop(labels=None, *, axis=0, index=None, columns=None, level=None, inplace=False, errors='raise')
Parameters:
labels:- A lable or column label in order to drop from the DataFrame.You can pass single label or single indices or list of lables or list of indices. axis:- {0 for index and 1 for column}, default is 0 or index.This parameter specifies whether to delete rows or columns. index:- Alternative to specify indices in order to delete. Remember, (labels, axis=0) is same as index=labels. columns:- Alternative to specify columns in order to delete. Remember, (labels, axis=1) is the same as columns=labels. level:- Optional, Int, or level name. It is used in MultiIndex DataFrame to specify which label should be removed. inplace:- Default is False, If it set to True then original DataFrame will be modified. errors:- It specify that how to handle errors if specified label or index not found in the Pandas DataFrame.By default it set to 'raise', that means KeyError will be raised if specify label or index will not found in the Pandas DataFrame.
This is all about the Pandas DataFrame drop() method, Now we are about to see multiple ways to form a column in Pandas DataFrame.
How to Remove a Single Column in Pandas DataFrame
As you can see in the above Pandas DataFrame, we have a total of four keys that are name, age, gender, and country but in the example section we are about to delete a country column from the Pandas DataFrame.
To delete the ‘country‘ column, pass country as the first parameter to the drop() method along with axis=1, If you have only one column to delete then you can pass that column name without a list or inside list but if you have more than one columns to delete you will have to pass all those columns inside the list.
Let’s delete the ‘country‘ column.
from pandas import DataFrame
dictionary = {
"name": ["Vishvajit", "Manish", "Ankit", "Harshita", "Manisha", "Aysha"],
"age": [25, 26, 21, 24, 23, 22],
"gender": ['Male', 'Male', 'Male', 'Female', 'Female', 'Female'],
"country": ['India', 'Australia', 'India', 'US', 'UK', 'India']
}
df = DataFrame(data=dictionary)
print("Original DataFrame")
print(df)
print("DataFrame after removing last column")
df = df.drop('country', axis=1)
print(df)
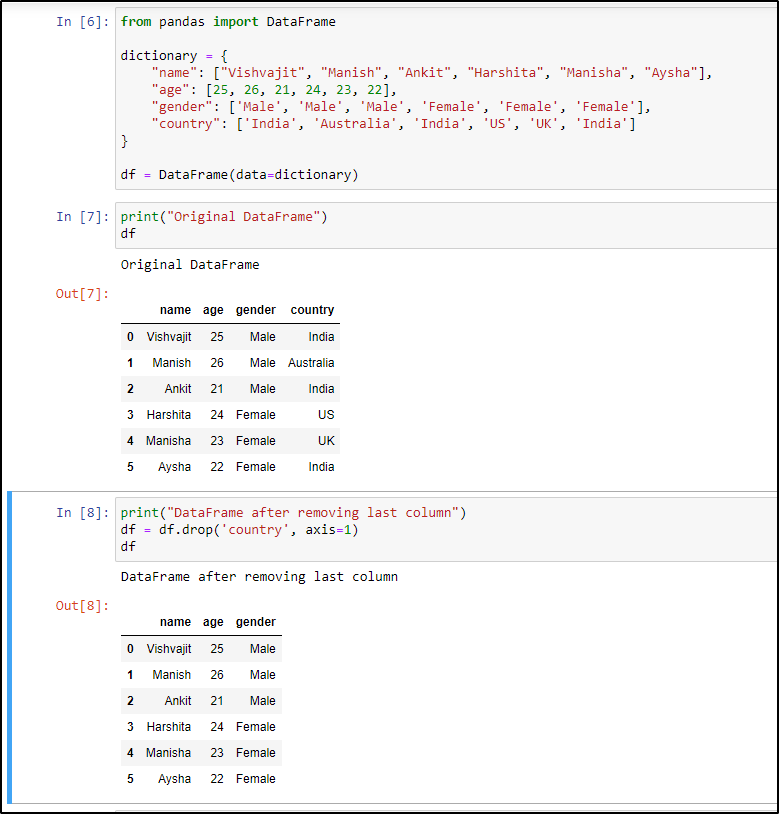
In the above example, I have used ‘country‘, axis=1 to delete the country column but you can also use columns=[‘country’] inside the drop() method in order to delete a single column from the pandas DataFrame.
How to delete Multiple Columns from Pandas DataFrame
There is no more complex code to delete multiple columns in Pandas DataFrame. We have to pass all the column names as a list that we want to drop to the first parameter of the drop() method.
For example, In the above-created sample DataFrame, I want to delete age and country, and then I will pass age and country in the list to the drop method as the first parameter along with axis=1.
from pandas import DataFrame
dictionary = {
"name": ["Vishvajit", "Manish", "Ankit", "Harshita", "Manisha", "Aysha"],
"age": [25, 26, 21, 24, 23, 22],
"gender": ['Male', 'Male', 'Male', 'Female', 'Female', 'Female'],
"country": ['India', 'Australia', 'India', 'US', 'UK', 'India']
}
df = DataFrame(data=dictionary)
print("Original DataFrame")
print(df)
print("DataFrame after removing last column")
df = df.drop(['age', 'country'], axis=1)
print(df)

You can also use columns=[‘age’, ‘country’] instead of [‘age’, ‘country’], axis=1 to delete multiple columns from the DataFrame.
How to delete the first column from Pandas DataFrame
To delete the first column from Pandas DataFrame, pass the first column name to the drop() method.
As you can see in the above DataFrame, the name is the first column and now I want to delete the ‘name’ column from the Pandas DataFrame, Then I will pass the name column to the drop method along with axis=1.
from pandas import DataFrame
dictionary = {
"name": ["Vishvajit", "Manish", "Ankit", "Harshita", "Manisha", "Aysha"],
"age": [25, 26, 21, 24, 23, 22],
"gender": ['Male', 'Male', 'Male', 'Female', 'Female', 'Female'],
"country": ['India', 'Australia', 'India', 'US', 'UK', 'India']
}
df = DataFrame(data=dictionary)
print("Original DataFrame")
print(df)
print("DataFrame after removing last column")
df = df.drop(['name'], axis=1)
print(df)

Pandas DataFrame drop() Method with inplace=True
The drop() takes a parameter inplace that ensures that the original DataFrame should be changed or not. If inplace=False then the drop() method will return a new DataFrame and if inplace= True then the drop() method will change the original Pandas DataFrame, By default incplce set to False.
let’s see drop() method with inplace=True.
from pandas import DataFrame
dictionary = {
"name": ["Vishvajit", "Manish", "Ankit", "Harshita", "Manisha", "Aysha"],
"age": [25, 26, 21, 24, 23, 22],
"gender": ['Male', 'Male', 'Male', 'Female', 'Female', 'Female'],
"country": ['India', 'Australia', 'India', 'US', 'UK', 'India']
}
df = DataFrame(data=dictionary)
print("Original DataFrame")
print(df)
print("DataFrame after deleteing first column")
df.drop('gender', axis=1, inplace=True)
print(df)
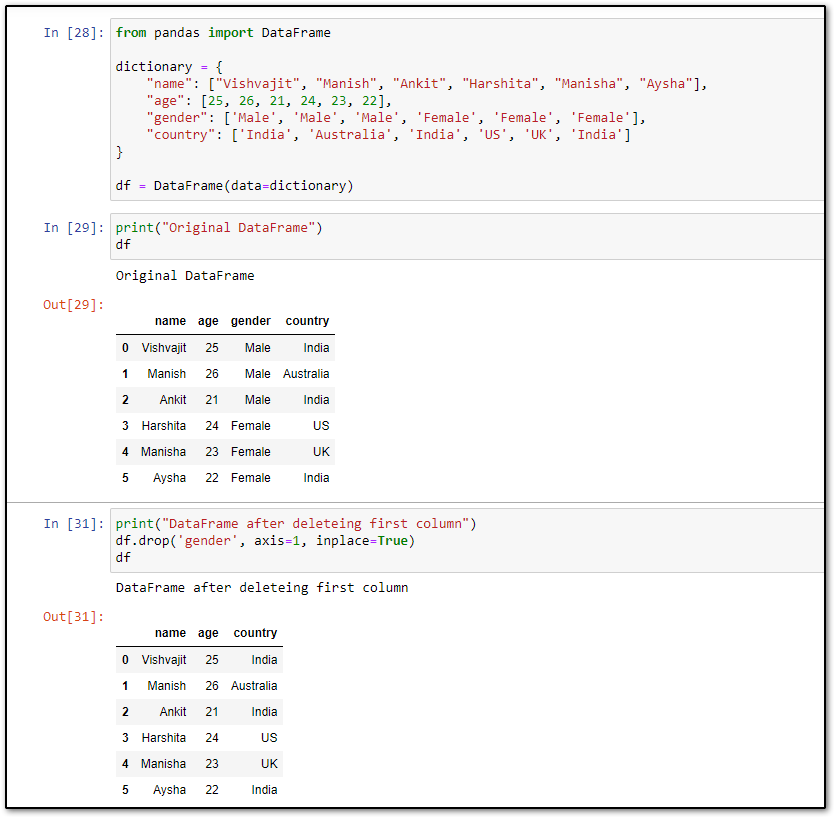
Conclusion
So throughout this article, we have seen how to delete column name from Pandas DataFrame with the help of the DataFrame drop() method. The drop() method is capable of deleting single or multiple columns from the Pandas DataFrame.
If you found this article helpful, please share and keep visiting for further Pandas tutorials.
Happy Coding…